Welcome to HBH! If you have tried to register and didn't get a verification email, please using the following link to resend the verification email.
File Searcher - Python Code Bank
File Searcher
Searches a directory and everything in it for a folder or file that has a name containing the keyword you give it.
from UserList import UserList
from os import listdir, curdir
from os.path import isfile, isdir
from os.path import join as joinpath
from os.path import split as splitpath
#version 0.7
class filespider:
def __init__(self,action,verbose=False):
self.verbose=verbose
self.action=action
def __scanpath(self,path):
if self.verbose:print "scanning:"+path
try:
items=listdir(path)
for item in items:
itempath=joinpath(path,item)
self.action(itempath)
if isdir(itempath):
if not itempath in self.pastpaths:
self.futurepaths.append(itempath)
except WindowsError:
print 'WindowsError encountered at:'+path
self.pastpaths.append(path)
def start(self,root):
self.pastpaths=[]
self.futurepaths=[]
if not isdir(root):
print "that's not a directory!"
return -1
print "scanning"
print root
self.futurepaths.append(root)
while self.futurepaths:
self.__scanpath(self.futurepaths.pop())
print 'scan complete'
class filterlist(UserList):#a list which is filtered by a given function
def __init__(self, filterfunction,initlist=None):
self.data = []
initlist=None
self.function=filterfunction
def append(self, item):
if self.function(item):
self.data.append(item)
def insert(self, i, item):
if self.function(item):
self.data.insert(i, item)
def isfoundin(keyword, string, capsense=False):
return keyword.lower() in string.lower()
rootpath=raw_input("Root path to begin searching at:")
keyword=raw_input("Keyword to search for:")
MyHits=filterlist(lambda x:isfoundin(keyword,splitpath(x)[-1]))
#note the use of lambda here. filterlist will pass one arguement,
#but isfoundin requires two. there must be a better way, but this works.
FileCrawler=filespider(MyHits.append)
FileCrawler.start(rootpath)
for i in MyHits:
print i
Comments
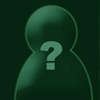
fuser 15 years ago
the script seems to work fine, not to mention quite fast in finding files according to the specified keywords, so congrats man.
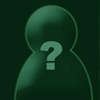