Secure Crypt - PHP Code Bank
php script:
///////////////////
<?php
if($_POST['task']!="" and $_POST['message']!="")
{
$message=$_POST['message'];
if($_POST['key']!="")
{
$key=md5($_POST['key']);
}
else
{
$key=md5('Long Text To Be Used As Default Key');
}
$array_key=str_split($key,1);
$array_final_key=array_unique($array_key);
foreach($array_final_key as $single_key)
{
$master_key[]=$single_key;
}
$counter_key=count($master_key);
if($_POST['task']!='decrypt')
{
// Encrypt
$message_array=str_split($message,$counter_key);
foreach($message_array as $chunk)
{
$single_char_value=str_split($chunk,1);
for($loop1=0;$loop1<$counter_key;$loop1++)
{
$ord_sum_char[]=ord($master_key[$loop1])+ord($single_char_value[$loop1]);
}
}
foreach($ord_sum_char as $to_encrypt)
{
$loop_val=intval($to_encrypt/11);
$mod_val=$to_encrypt%11;
switch($mod_val)
{
case "0";
$char_fixed_val='<';
break;
case "1";
$char_fixed_val='*';
break;
case "2";
$char_fixed_val='$';
break;
case "3";
$char_fixed_val='#';
break;
case "4";
$char_fixed_val='+';
break;
case "5";
$char_fixed_val='?';
break;
case "6";
$char_fixed_val='>';
break;
case "7";
$char_fixed_val='^';
break;
case "8";
$char_fixed_val='!';
break;
case "9";
$char_fixed_val='&';
break;
case "10";
$char_fixed_val='%';
break;
}
$encryptd_char[]=$loop_val.$char_fixed_val;
}
$final_message=implode(" ",$encryptd_char);
echo'<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<title>
Super Crypt By ADIGA
</title>
<meta http-equiv="content-type" content="text/html; charset=UTF-8">
<link rel="stylesheet" type="text/css" href="style.css">
</head>
<body>
<div id="main_container">
<div id="body_container">
<div id="header">Super Crypt</div>
<form method="post" action="index.php">
<textarea name="message" id="text_area">'.$final_message.'</textarea>
<select name="task" id="task">
<option value="encrypt">Encrypt Text</option>
<option value="decrypt">Decrypt Text</option>
</select>
<input type="submit" value="Encrypt/Decrypt" id="button">
<div id="key">Key :</div>
<input type="text" name="key" id="key_text">
</form>
</div>
</div>
</body>
</html>';
}
else
{
// Decrypt
$first_decrypt_array=explode(" ",$message);
$counter_element=0;
$text_reset="";
foreach($first_decrypt_array as $first_value)
{
$counter_element=$counter_element+1;
if($counter_element%$counter_key=="0")
{
$text_reset=$text_reset.$first_value."Q";
}
else
{
$text_reset=$text_reset.$first_value." ";
}
}
$text_fixed=rtrim($text_reset,"Q");
$array_sectors=explode("Q",$text_fixed);
foreach($array_sectors as $sector)
{
$array_chars_fixed=explode(" ",$sector);
$counter_chars_fixed=count($array_chars_fixed);
for($loop_fixed=0;$loop_fixed<$counter_chars_fixed;$loop_fixed++)
{
$char_single_fixed=substr($array_chars_fixed[$loop_fixed],-1);
$loops_fixed=str_replace($char_single_fixed,"",$array_chars_fixed[$loop_fixed]);
$loops_fixed_val=$loops_fixed*11;
switch($char_single_fixed)
{
case "<";
$char_fixed_val=0;
break;
case "*";
$char_fixed_val=1;
break;
case "$";
$char_fixed_val=2;
break;
case "#";
$char_fixed_val=3;
break;
case "+";
$char_fixed_val=4;
break;
case "?";
$char_fixed_val=5;
break;
case ">";
$char_fixed_val=6;
break;
case "^";
$char_fixed_val=7;
break;
case "!";
$char_fixed_val=8;
break;
case "&";
$char_fixed_val=9;
break;
case "%";
$char_fixed_val=10;
break;
}
$final_val=($loops_fixed_val+$char_fixed_val)-ord($master_key[$loop_fixed]);
if($final_val!=0)
{
$decoded_message[]=chr($final_val);
}
}
}
$final_message1=implode("",$decoded_message);
$fh=fopen("Decoded.txt","w+");
fputs($fh,$final_message1);
fclose($fh);
echo'<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<title>
Super Crypt By ADIGA
</title>
<meta http-equiv="content-type" content="text/html; charset=UTF-8">
<link rel="stylesheet" type="text/css" href="style.css">
</head>
<body>
<div id="main_container">
<div id="body_container">
<div id="header"><a href="Decoded.txt">Decoded Message</a></div>
</div>
</div>
</body>
</html>';
}
}
else
{
echo '<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<title>
Super Crypt By ADIGA
</title>
<meta http-equiv="content-type" content="text/html; charset=UTF-8">
<link rel="stylesheet" type="text/css" href="style.css">
</head>
<body>
<div id="main_container">
<div id="body_container">
<div id="header">Super Crypt</div>
<form method="post" action="index.php">
<textarea name="message" id="text_area"></textarea>
<select name="task" id="task">
<option value="encrypt">Encrypt Text</option>
<option value="decrypt">Decrypt Text</option>
</select>
<input type="submit" value="Encrypt/Decrypt" id="button">
<div id="key">Key :</div>
<input type="text" name="key" id="key_text">
</form>
</div>
</div>
</body>
</html>';
}
?>
///////////////////
style file (style.css)
///////////////////
html{
cursor: default;
}
body{
margin: 0px;
font-size: 14px;
color: #ff0000;
font-weight: bold;
background: #000000;
}
#main_container{
width: 500px;
margin-left: auto;
margin-right: auto;
}
#body_container{
width: 500px;
height: 600px;
position: relative;
top: 0px;
left: 0px;
}
#header{
height: 50px;
width: 498px;
background: #000000;
position: absolute;
top: 100px;
left: 0px;
padding-top: 5px;
padding-left: 0px;
padding-right: 0px;
padding-bottom: 0px;
font-size: 32px;
color: #ff0000;
text-align: center;
border: 1px solid #ff0000;
}
#text_area{
height: 300px;
width: 500px;
border: 0px;
background: #ff0000;
position: absolute;
top: 160px;
left: 0px;
cursor: default;
font-size: 14px;
font-weight: bold;
}
#task{
width: 110px;
position: absolute;
top: 470px;
left: 0px;
background: #ff0000;
border: 0px;
}
#button{
width: 110px;
position: absolute;
top: 470px;
left: 120px;
background: #ff0000;
border: 0px;
}
#key{
position: absolute;
top: 470px;
left: 255px;
}
#key_text{
width: 200px;
background: #ff0000;
position: absolute;
top: 470px;
right: 0px;
border: 0px;
}
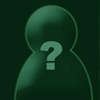
ghost 13 years ago
While I do like the method you went about creating your own encryption/decryption script, there are multiple places where you can make it more efficient (as you have said in your description). I understand what you are doing just messing around with it though (instead of focusing on efficiency) which is totally fine and good :-).
I do question, however, your use of "array_unique". Why would you want to do that? Because of that, any key of "abc" will also encrypt/decrypt the same as "aaaabbbbccccc" (at least according to my reading of your script…I haven't actually run it). Is there a reason you added that line? I'd like to know.
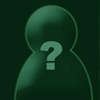
ADIGA 13 years ago
the key it self is not used directly, its the unique chars in its md5. md5(abc) != md5(aaaabbbccc).
the idea is to make the number of chars used to encrypt the text harder to guess.