Maths, Multiply any numbers. - PHP Code Bank
/*A function that takes 2 numbers and multiply them, capable of multiplying very large numbers.*/
Function Mul($NumA, $NumB){/*Takes 2 numbers, not picky what kind, but strings generally work better.*/
$Num1=Str_Split($NumA); $Num2=Str_Split($NumB);/*It then splits the numbers to arrays, a digit a cell.*/
ForEach ($Num2 as $N2){++$Down;$Across=-1;/*We loop the numbers of 1 array, inc 1 counter, resetting another.*/
ForEach ($Num1 as $N1){++$Across;/*We loop through the second number, increasing the counter that gets reset.*/
$Sum[$Down+$Across]+=$N1*$N2;/*Save the result of multiplying the 2 cells, saving the result to a new array.*/
}
}
$Sum=Array_Reverse($Sum);/*For ease we reverse the array.*/
ForEach ($Sum as $Digit){ ++$Finish;/*And start going through each cell, and counting it.*/
$Add=$Digit+$Carry;/*The number we need is the cell digit plus carry over, if any.*/
If ($Add>=10){/*If the result is above 9, or 2 digits long, we have carry over.*/
$Total[$Finish]=$Add%10;/*We use the last digit for our final answer.*/
$Carry=$Add/10-(Total[$Finish]/10);}/*And carry over the tens/hundreds as units/tens etc.*/
Else{
$Total[$Finish]=$Add;/*Else we add on the total.*/
$Carry=0;}/*The carry over is 0.*/
}
Array_Push($Total,$Carry);/*To get the final number we need to add on any left over 'carry'.*/
$Total=Array_Reverse($Total);/*Reverse the whole thing once again.*/
$Total=Implode($Total);/*Implode it.*/
$Total=LTrim($Total,"0");/*And trim of the left hand 0's.*/
Return $Total;/*Done.*/
}
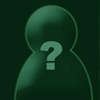
ghost 16 years ago
Your coding still reeks of inconsistency. You initialize one array ($row), but you fail to initialize the $ch array. $x = $x + 2 would be equivalent to the shorthand $x += 2 (line 28). Your coding is mostly competent, but your comments show us that you're not entirely sure why. You're doing some quality things in this code; now, you need to polish it to the point where we, as willing critics, do not have to wonder whether you know what you're doing or not. Great job, but I'm thinking you're capable of more. I'm only giving out a "Good" on this one because I think you need something to motivate you to do something more "stable".
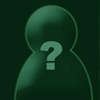
K3174N 420 16 years ago
I couldnt agree more Z, thanks for that. I am by no means an expert to php, by no means… I made this to learn more on arrays really, learn a few new styles… But what was that about line 28… "$ch[$i+$d]=$ch[$i+$d]+array_shift($row);".. wheres the +2? its $i+$d… I have posted this on php freaks, and have been told about a few commands im using which are long, and a few more efficiant ways to do things, this code started as a shambled mess, its not perfect yet, but im getting closer :)
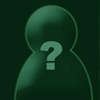
ghost 16 years ago
Progress is a constant state, and it's obvious that you're making it. Also, there have been MUCH worse code submissions; this is actually an above-average one. I specifically pointed out line 28 when mentioning the shorthand because I didn't feel like typing it out, so I used the +2 as an example. You're doing $ch[$i+$d] = $ch[$i+$d] + array_shift($row), when you could just be doing $ch[$i+$d] += array_shift($row). Oh, and unless there's some particular reason you're initializing the array with a value of 0 for the first key, you can just initialize an array by setting it equal to array(). Good luck with the improvements.
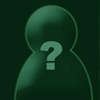
K3174N 420 16 years ago
ahh right, see its the shorthand stuff like += that i dont know ^^ And for the array, yea, i suppose 0=>0 is a bit pointless… Ideally i would like to just initialize it once… Cheers for that.
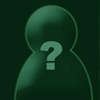
K3174N 420 16 years ago
Ok, i thin its finished, i see no way to make it shorter now… It is also the fastest version so far.
function mul($numa, $numb){
$arr1=str_split($numa); $arr2=str_split($numb);
foreach ($arr2 as $d){ ++$down; $across=-1;
foreach ($arr1 as $i){ ++$across;
$ch[$down+$across]+=$i*$d;
}
}
$ch=array_reverse($ch);
foreach ($ch as $i){ ++$fin;
$add=$i+$carry;
if ($add>9){
$total[$fin]=substr($add,-1,1);
$carry=($add-$total[$fin])/10;
}
else{
$total[$fin]=$add;
$carry=0;
}
}
array_push($total,$carry); $total=array_reverse($total);
$total=implode($total); $total=ltrim($total,"0");
return $total;
}
```:ninja::ninja:
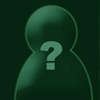
K3174N 420 16 years ago
Still no replys :(
Anyway, replace:
$total[$fin]=substr($add,-1,1);
$carry=($add-$total[$fin])/10;
with:
$total[$fin]=$add%10;
$carry=$add/10-($total[$fin]/10);
Quicker, no string funtions.
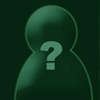
ghost 16 years ago
Amazing how simple and efficient code makes for the fastest… eh? :P Nice job. Optimizing code is an art.