Base value 62 - PHP Code Bank
function increment_base62($num)
{
$z=0; //keeps count of the Z's
$count=strlen($num); //Checks length of the number
$array=str_split($num); //splits the number to an array
for ($i=$count;$i>0;$i--){ //loops $count down to 1.
if ($array[$i-1]=='Z') //if the char is a Z...
$z++; //add to the Z counter
}
if ($z==$count) //if the Z counter is the same as the number length (all Z's)
$num=str_repeat('0',$count+1); //Set the new number as a longer one of 0's
else{ //else we start incrementing....
for ($i=$count;$i>0;$i--){ //another loop that loops $count down to 1.
if ($array[$i-1]!='Z'){ //this will skip all the Z's till we get a char we can increment
if ($array[$i-1]=='9') //if its a 9,
$array[$i-1]='a'; //we increment it to an 'a'
elseif ($array[$i-1]=='z') //else if its a z
$array[$i-1]='A'; we increment it to an 'A'
else{
$chr=ord($array[$i-1]); //else we grab its ascii value
$array[$i-1]=chr($chr+1); //and set it as the next ascii char up.
}
if ($i<$count){ //if it wasn't the last number, we need to set 0's
for ($s=$i;$s<$count;$s++){ //this loops how many numbers in we are...
$array[$s]=0; //and clocks the end numbers to 0.
}
}
$num=implode($array); //we can now stick the array back together
$i=-1; //exit the loop
}
}
}
return $num; //and return the incremented value.
}
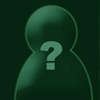
K3174N 420 16 years ago
Gah! typo on line 18… missed // >.< At least this new code bank makes it obvious…
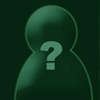
ghost 16 years ago
The first loop to find 'Z' could've just been $z = preg_match_all("/Z/",$num), since preg_match_all returns the number of occurrences in a string. Also, the repeated for's and conditionals should be a switch and (hopefully) a single for. The fact that you are breaking out of one of your for loops by setting the variable outside of the range should tell you that something is less than ideal. Don't be afraid to use multiple return statements in a function… they make sense when you've got nothing left to do in the function. Returns would be ideal at the $z == $count conditional and the $i = -1 assignment. Not sure of any cases in which I'd actually use this kind of code, but you put some effort into it. Looking forward to your next submission after this unique one.
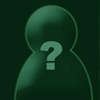
K3174N 420 16 years ago
Cheers for the feedback Zeph, your right for the fact that is a preg_match statement even i can understand ^^. As for the returns, good call, and tbh never occurred to me… >.< Edited on my site, anyone here will have to take your advice on their own there. It probably isn't a function most people would need, but i made it because i did need it, and your right when you mentioned i put a lot of thought into it. Was happy when i got it working :) So i saw no harm in sticking it up here ;)
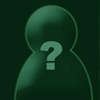
ghost 16 years ago
Yeah, there was no harm in putting it here… interesting code belongs here, and this was. If you revised the source, just post the revisions as a comment here so that everyone can reference them in place. :)
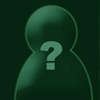
K3174N 420 16 years ago
heres a quick copy of a newer version using Z's advice. Works well :)
function increment_base62($num)
{
$count=strlen($num);
$array=str_split($num);
if (preg_match_all("/Z/",$num, $matches)==$count){
$num=str_repeat('0',$count+1);
return $num;
}
for ($i=$count;$i>0;$i--){
if ($array[$i-1]!='Z'){
switch ($array[$i-1])
{
case '9':
$array[$i-1]='a';
break;
case 'z':
$array[$i-1]='A';
break;
default:
$chr=ord($array[$i-1]);
$array[$i-1]=chr($chr+1);
}
if ($i<$count){
for ($s=$i;$s<$count;$s++){
$array[$s]=0;
}
}
$num=implode($array);
return $num;
}
}
return $num;
}
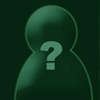