Shell like program - Perl Code Bank
#!/usr/bin/perl
#Created by TheSilentDrifter 02/25/2009 | 6:47 MST#
use strict;
my($user, $pass, $input);
sub help
{
print "cd | cat | ls\/dir | mkdir | quit | touch | chmod | rm | edit\n";
}
sub cat
{
my($file_o, @o_file);
print "cat: What file would you like to open?: ";
chomp($file_o = <STDIN>);
if(-e $file_o)
{
open FILE, "<$file_o" or warn "cat: Error opening file!: $!\n";
@o_file = <FILE>;
foreach (@o_file)
{
print "$_\n";
}
}
else
{
print "cat: Could not open file because: $!\n";
}
}
sub mk_dir
{
my($new_dir, $input);
print "mkdir: What would you like to call the new folder?: ";
chmod($new_dir = <STDIN>);
if(-e $new_dir)
{
print "mkdir: Warning! This folder exists! Would you like to overwrite it?: [YES/no]";
chomp($input = <STDIN>);
if($input eq "" || ($input =~ m/\byes\b/i || m/\by\b/i))
{
mkdir $new_dir, 0755 or warn "mkdir: Could not make $new_dir: $!\n";
}
else
{
print "mkdir: Ok, the directory will not be created.\n";
}
}
else
{
mkdir $new_dir, 0755 or warn "Could not make $new_dir: $!\n";
}
}
sub touch
{
my($file, $input);
print "touch: Insert the name of the file you wish to create: ";
chomp($file = <STDIN>);
if(-e $file)
{
print "touch: " . $file . " exists. Would you like to overwrite it?: [YES/no]";
chomp($input = <STDIN>);
if(($input =~ m/\byes\b/i || $input=~ m/\by\b/i) || $input eq "")
{
open(FILE, ">$file") or warn "touch: Could not create file: $!\n";
}
else
{
print "touch: File will not be created.\n";
}
}
else
{
open(FILE, ">$file") or warn "touch: Could not create file: $!\n";
}
}
sub dir
{
my($dir, $num, @dir);
sub n
{
$num += 1;
}
@dir = glob "*";
print "\nFiles in current directory: \n\n";
foreach $dir (@dir)
{
print "\t(" . &n . ") " . $dir . "\n\n";
}
}
sub ch_mod
{
my($file, $file_perm);
print "chmod: What file should have permissions changed on it?: ";
chomp($file = <STDIN>);
print "chmod: What permissions should " . $file . " have?: ";
chomp($file_perm = <STDIN>);
oct($file_perm);
if ($file_perm eq "0644" or $file_perm eq "644")
{
chmod(0644, $file) or warn "chmod: " . $file . "'s permissions were not changed!: $!\n";
}
elsif ($file_perm eq "0755" or $file_perm eq "755")
{
chmod(0755, $file) or warn "chmod: " . $file . "'s permissions were not changed!: $!\n";
}
elsif ($file_perm eq "0777" or $file_perm eq "777")
{
chmod(0777, $file) or warn "chmod: " . $file . "'s permissions were not changed!: $!\n";
}
else
{
warn "chmod: " . $file . "'s permissions were not changed because you did not enter a permission level!\n";
}
}
sub cd
{
my($dir, $input);
print "cd: Where would you like to go?: ";
chomp($input = <STDIN>);
chdir $dir or warn "cd: Cannot change to directory: $!\n";
}
sub rm
{
my($file);
print "rm: What file would you like to remove?: ";
chomp($file = <STDIN>);
if (-e $file)
{
print "rm: Are you sure you want to delete the file?: [YES/no]";
chomp($input = <STDIN>);
if ($input eq "" || ($input =~ /\byes\b/i || $input =~ /\by\b/i))
{
unlink $file or warn "rm: " . $file . " could not be removed: $!\n";
print "rm: " . $file . " was removed.\n";
}
else
{
print "rm: The file will not be removed then.\n";
}
}
else
{
warn "rm: " . $file . "Could not be removed!: $!\n";
}
}
sub edit
{
my($old_file, $new_file);
print "edit: What file would you like to edit?: ";
chomp($old_file = <STDIN>);
if (-e $old_file)
{
my($orig_word, $new_word, @edit, $line);
print "edit: What word in \"" . $old_file . "\" would you like to edit?: ";
chomp($orig_word = <STDIN>);
print "edit: What would you like to replace \"" . $orig_word . "\" with in \"" . $old_file . "\"?: ";
chomp($new_word = <STDIN>);
open(OLD, "<$old_file") || warn "edit: Could not open file: $!\n";
$new_file = "$old_file~";
open(NEW, ">>$new_file") || warn "edit: Could not open file: $!\n";
while(<OLD>)
{
$line = s/\b$orig_word\b/$new_word/ig;
if($line)
{
print NEW "$_";
print "edit: \"" . $orig_word . "\" was replaced with \"" . $new_word . "\".\n";
close(OLD);
close(NEW);
rename $new_file, $old_file || die "edit: Error replacing original file: $!\n";
}
else
{
print "edit: Could not replace \"" . $orig_word . "\": $!\n";
close(OLD);
close(NEW);
}
}
}
}
sub re_name
{
my($old_name, $new_name);
print "rename: What file would you like to rename?: ";
chomp($old_name = <STDIN>);
if (-e $old_name)
{
print "rename: What should \"" . $old_name . "\" be changed to?: ";
chomp($new_name = <STDIN>);
if( -e $new_name)
{
warn "rename: Cannot rename to \"" . $new_name . "\": It already exists!\n";
}
else
{
rename $old_name, $new_name || warn "rename: Could not rename \"" . $old_name . "\": $!\n";
print "rename: \"" . $old_name . "\" is now named \"" . $new_name . "\". \n";
}
}
else
{
warn "rename: Could not rename file: $!\n";
}
}
do
{
print "Users\@Shell> ";
chomp($input = <STDIN>);
SWITCH:
{
if($input eq "help") {help(); last SWITCH;}
if($input eq "cat") {cat(); last SWITCH;}
if($input eq "cd") {cd(); last SWITCH;}
if($input eq "mkdir") {mk_dir(); last SWITCH;}
if($input eq "touch") {touch(); last SWITCH;}
if($input eq "chmod") {ch_mod(); last SWITCH;}
if($input eq "rm" ) {rm(); last SWITCH;}
if($input eq "edit") {edit(); last SWITCH;}
if($input eq "rename") {re_name(); last SWITCH;}
if($input eq "ls" || $input eq "dir") {dir(); last SWITCH;}
}
}
until (($input =~ /quit/i) || ($input =~ /exit/i));
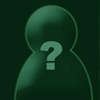
clone4 15 years ago
even though it is kind unique, some of the techniques you use are out dated, and the whole purpose of this script is, well little strange to say the least, because practically what is the use:)…?
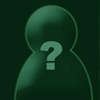
TheSilentDrifter 15 years ago
well, overall the purpose was just to see if i could do it. I'm still a beginner to perl, so it's the best i could come up with. Wouldn't mind a few pointers, though. Any ideas to improve it?
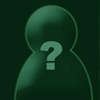
clone4 15 years ago
okay, I went through the code in more detail and honestly it's full of bad coding practice. Here I tried to just for example correct first two subroutines (it's late and I'm tired, so there might be some mistakes):
sub cat { my($file_o); print "cat: What file would you like to open?: "; chomp($file_o = <STDIN>); if(-e $file_o) { open $file, "$file_o" or warn "cat: Error opening file!: $!\n"; while(<$file>) { print "$_"; } } else { print "cat: Could not open file because: $!\n"; } }
sub mk_dir { my($new_dir, $input); print "mkdir: What would you like to call the new folder?: "; chmod($new_dir = <STDIN>); if(-e $new_dir) { print "mkdir: Warning! This folder exists! Would you like to overwrite it?: [YES/no]"; chomp($input = <STDIN>); if($input =~ /(yes|y)/i) { mkdir $new_dir, 0755 or warn "mkdir: Could not make $new_dir: $!\n"; } else { print "mkdir: Ok, the directory will not be created.\n"; } } else { mkdir $new_dir, 0755 or warn "Could not make $new_dir: $!\n"; } }
if you need more help just hit me up with pm
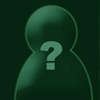
clone4 15 years ago
oops sorry for the format, forgot we aren't in forum:) still should give you rough idea where to look;)
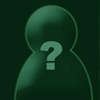
TheSilentDrifter 15 years ago
sweet, thanks clone. I'll take a look at it when i get a chance. I also forgot to change the edit subroutine, too. I have the variable @edit defined and not being used like it should be. That line should use foreach instead of a while loop. Anyway, thanks again.