Welcome to HBH! If you have tried to register and didn't get a verification email, please using the following link to resend the verification email.
add group - Java Code Bank
add group
this little program allow you to create a group, see who is in this group.
/*
* File: Person.java.
* This Program just set up the attribute needed for person.
*/
import java.util.*;
public class Person {
private String firstName;
private String LastName;
private int personAge;
public Person(String name, String Lastname, int age){
firstName = name;
LastName = Lastname;
personAge = age;
}
public String getFirstName(){
return firstName;
}
public String getLastName(){
return LastName;
}
public void setAge(int age){
personAge = age;
}
public int getAge(){
return personAge;
}
public String toString(){
return (firstName + " " + LastName + " is " + personAge);
}
}
--------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
/*
* File: Group.java
* This code just set up the attribute needed for a group.
*/
// THis is the import needed
import java.util.*;
public class Group {
// constructor with Parameters.
public Group(String groupName, String leader){
group = groupName;
Leader = leader;
}
// The get group method that get the group
public String getGroup(){
return group;
}
// this just get the leader's name.
public String getLeader(){
return Leader;
}
// This methods just add people to the arraylist.
public void addPeople(Person people1){
people.add(people1);
}
// This method return people as an iterator.
public Iterator<Person> getPeople(){
return people.iterator();
}
// The toString method.
public String toString(){
return ("\"" + group + "\"" + "was added by: " + Leader);
}
// Private Instances variable.
private String group;
private String Leader;
ArrayList<Person> people = new ArrayList<Person>();
}
--------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
/*
* File : Socialnetworks.java
* **************************************************
* This program allow you to create group of people.*
* it allow you to see people in a particular group.*
* Created by buddywithgol *
* v.1.0.0.0.0..0 *
*/
// You need to download the acm library for this program.
import acm.program.*;
import java.util.*;
public class SocialNetworks extends ConsoleProgram{
// This is the run method which basically run everything in your program.
public void run(){
// The while loop run until the user tell it to stop. Which is to input 0.
while(true){
int selection = getSelection();
if(selection ==QUIT)break;
// This is the switch case that let the user to decide what kind of action he/she want to make.
switch(selection){
case LIST_PEOPLE:
listPeople();
break;
case LIST_GROUP:
listGroup();
break;
case ADD_PERSON:
addPeople();
break;
case ADD_GROUP:
addGroup();
break;
case LIST_PEOPLE_ON_GROUP:
listPeopleInAGroup();
break;
case UPDATE_PEOPLE_AGE:
}
}
}
// This method get the selection from the user. It read an integer to decide what kind of action to be execute.
private int getSelection(){
println("");
println("Press 0 to Quit");
println("Enter 1 to list Person in a group");
println("Enter 2 to list the available group");
println("Enter 3 to add a person to a group");
println("Enter 4 to create a group");
println("Enter 5 to list the people on a particular group");
println("Enter 6 to update a person's age");
int select = readInt("Selection:");
return select;
}
// When this method is called it list all people in the database. Not for a particular group.
private void listPeople(){
// This goes through every index of the ArrayList and print them out on the screen.
for(int i = 0;i<People.size();i++){
println(People.get(i).toString());
}
}
private void listGroup(){
Iterator<String> groupIt = Group.keySet().iterator();
while(groupIt.hasNext()){
println(Group.get(groupIt.next()).toString());
}
}
private Person addPeople(){
String name = readLine("Person's first name (Enter to quit): ");
if (name.equals("")) return null;
String band = readLine("Last name: ");
int songIndex = findPerson(name, band);
if (songIndex != -1) {
println("That song is already in the store.");
return People.get(songIndex);
} else {
int price = readInt("Age: ");
Person song = new Person(name, band, price);
String group = readLine("Group: ");
People.add(song);
println("New Person added to the store.");
return song;
}
}
private int findPerson(String name, String group){
int index = -1;
for(int i = 0; i<People.size();i++){
if(People.get(i).getFirstName().equals(name)
&& People.get(i).getLastName().equals(group)){
index = i;
break;
}
}
return index;
}
private void addGroup(){
String name = readLine("Group name:");
if(Group.containsKey(name)){
println("This Group is already. Please choose another name for your group.");
}else{
String leader = readLine("leader's name");
Group group = new Group(name,leader);
Group.put(name, group);
while(true){
Person person = addPeople();
if(person == null)break;
group.addPeople(person);
}
println("New group created by " + leader);
}
}
private void listPeopleInAGroup(){
String name = readLine("Group Name: ");
if(Group.containsKey(name)){
Iterator<Person> peopleIT = Group.get(name).getPeople();
println(name + " contains the following People: ");
while (peopleIT.hasNext()){
Person person = peopleIT.next();
println(person.toString());
}
}
}
private static final int QUIT = 0;
private static final int LIST_PEOPLE = 1;
private static final int LIST_GROUP = 2;
private static final int ADD_PERSON = 3;
private static final int ADD_GROUP = 4;
private static final int LIST_PEOPLE_ON_GROUP = 5;
private static final int UPDATE_PEOPLE_AGE = 6;
// This store all the group into a Hashmap
private HashMap<String,Group> Group = new HashMap<String,Group>();
// This arrayList store Evryone that was added
private ArrayList<Person> People = new ArrayList<Person>();
}
Comments
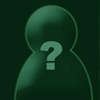