Welcome to HBH! If you have tried to register and didn't get a verification email, please using the following link to resend the verification email.
number-to-word converter - Java Code Bank
number-to-word converter
a program that takes a number as input from the user and converts it into words and displays it
public class word_converter_fn
{
public static String convert(int n) // this function converts numbers from 1 to 99 into words
{
String out = "";int flag = 0;
String tens[] = {"ten","twenty","thirty","forty","fifty","sixty","seventy","eighty","ninety"};
String elevens[] = {"eleven","twelve","thirteen","fourteen","fifteen","sixteen","seventeen","eighteen","nineteen"};
String ones[] = {"one","two","three","four","five","six","seven","eight","nine"};
if (n >= 10)
{
if(n < 20 && n > 10)
{
out += " " + elevens[(n%10)-1];
flag = 1;
}
else
{
out += " " + tens[(n/10)-1];
n = n%10;
}
}
if(n < 10 && n > 0 && flag == 0)
{
out += " " + ones[n-1];
}
return out;
}
/* enter no. to be written in words (less than 1 crore)*/
public static void main(int i) // this function takes input from user , sends it to convert() and adds suffixes like lakh, thousand ,etc.
{
int a = i;
String dis = "";
if(a == 0)
dis = "zero" ;
if(a > 100000)
{
int x = (int)a/100000;
dis = dis + convert(x) + " lakh";
a = a % 100000;
}
if(a >= 1000 && a < 100000)
{
int x = (int)a/1000;
dis = dis + convert(x) + " thousand";
a = a % 1000;
}
if(a >= 100 && a < 1000)
{
int x = (int)a/100;
dis = dis + convert(x) + " hundred";
a = a % 100;
}
if(a >= 0 && a < 100)
dis = dis + convert(a);
dis = dis.trim();
System.out.println("input : " + i);
System.out.println("Output : " + dis);
}
}
Comments
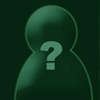
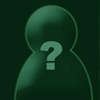
newbee 13 years ago
for those who are having problems executing it ,
-
change public static void main(int i) to public static void main(String args[])
-
insert these lines after the declaration of main method :-
Scanner sc = new Scanner(System.in);
System.out.println("Enter any no.");
int i = sc.nextInt();