Welcome to HBH! If you have tried to register and didn't get a verification email, please using the following link to resend the verification email.
IRC Bot - Java Code Bank
IRC Bot
A bot I've been working on lately.
Just so you know, this requires that you download PIRCBot ( http://www.jibble.org/pircbot.php ) to run it.
Some suggestions or comments would be nice.
TO ADD:
-more functions to the bot itself
-a swing interface
Oh, and the MD5 thing does have some relevance. At least, it will soon.
import java.util.*;
import java.util.regex.*;
import java.text.*;
import java.io.*;
import java.nio.*;
import org.jibble.pircbot.*;
import java.security.*;
import java.net.*;
interface CommandAction {
public Vector action(String[] args, HashMap cData);
public String help();
public boolean priv();
}
class MD5 {
public String MD5(String out) {
String ret = "";
try{
MessageDigest algorithm = MessageDigest.getInstance("MD5");
algorithm.reset();
algorithm.update(out.getBytes());
byte messageDigest[] = algorithm.digest();
StringBuffer hexString = new StringBuffer();
for (int i=0;i<messageDigest.length;i++) {
String hex = Integer.toHexString(0xFF & messageDigest[i]);
if(hex.length()==1)
hexString.append('0');
hexString.append(hex);
}
ret = hexString.toString();
} catch(NoSuchAlgorithmException nsae){
ret = null;
nsae.printStackTrace();
}
return ret;
}
}
class Bot extends PircBot {
private static Map cmdMap;
private static String host;
private static int port;
private static String sqlHost, sqlUser, sqlPass, sqlDB, admin;
private static boolean coding;
private static String code, name, t;
public Bot() {
Scanner kbd = new Scanner(System.in);
System.out.print("Your nick: ");
admin = kbd.nextLine();
System.out.print("Bot name: ");
String n = kbd.nextLine();
this.setName(n);
System.out.print("Host: ");
host = kbd.nextLine();
System.out.print("Port: ");
port = kbd.nextInt();
cmdMap = new HashMap();
this.setVerbose(true);
this.setVersion((new Double(1.0)).toString());
this.setMessageDelay(1);
}
public String getAdmin() {
return admin;
}
public boolean isOp(String name, String ch) {
User[] users = this.getUsers(ch);
for(User u : users)
if(u.getNick().equals(name))
return u.isOp();
return false;
}
public boolean isOp(String ch) {
return isOp(this.getAdmin(),ch);
}
public boolean isValid(String n) {
return n.equals(admin);
}
public String[] parse(String msg) {
return msg.substring(1).split(" ");
}
public String[] args(String[] cArr) {
String[] ret;
if(cArr.length>1) {
ret = new String [cArr.length-1];
for(int i = 1; i < cArr.length; i++) {
ret[i-1]=cArr[i];
}
} else {
ret = null;
}
return ret;
}
public void connect() {
try { this.connect(host,port); }
catch (Exception e) { e.printStackTrace(); }
}
public void join(String Channel) {
this.joinChannel("#"+Channel);
}
public void part(String Channel, String Reason) {
this.partChannel("#"+Channel,Reason);
}
public boolean inChan(String chan) {
String[] chans = this.getChannels();
for(int i = 0; i < chans.length; i++)
if(chans[i].equals("#"+chan))
return true;
return false;
}
protected void onNickChange(String oldNick, String login, String hostname, String newNick) {
if(oldNick.equals(admin)) {
admin = newNick;
}
}
public void onVersion(String sourceNick, String sourceLogin, String sourceHostname, String target) {
if(target.equals(this.getNick())) {
this.sendMessage(sourceNick, "VERSION "+this.getVersion());
}
}
public void onPrivateMessage(String sender, String login, String hostname, String message) {
if(!message.startsWith("$")) {
return;
}
String[] cArr = parse(message);
String cmd = cArr[0].toUpperCase();
String[] cArgs = args(cArr);
int len = 0;
try {
len = cArgs.length;
} catch (Exception e) {
len = 0;
}
Integer l = new Integer(len);
CommandAction cAct;
Vector rMsg = new Vector();
if((cAct = ((CommandAction)cmdMap.get(cmd))) != null) {
HashMap cData = new HashMap();
cData.put("sender",sender);
cData.put("login",login);
cData.put("hostname",hostname);
cData.put("message",message);
cData.put("type","onPrivateMessage");
cData.put("cmdMap",cmdMap);
cData.put("args",l);
rMsg = cAct.action(cArgs, cData);
}
else { }
if(rMsg == null) { return; }
for(int i = 0; i < rMsg.size(); i++) {
this.sendMessage(sender,(String)rMsg.get(i));
}
}
public String getReason() {
return "OH NOES.";
}
public void onMessage(String channel, String sender, String login, String hostname, String message) {
if(!message.startsWith("$")) {
return;
}
String[] cArr = parse(message);
String cmd = cArr[0].toUpperCase();
String[] cArgs = args(cArr);
int len = 0;
try {
len = cArgs.length;
} catch (Exception e) {
len = 0;
}
Integer l = new Integer(len);
CommandAction cAct;
Vector rMsg = new Vector();
if((cAct = ((CommandAction)cmdMap.get(cmd))) != null) {
HashMap cData = new HashMap();
cData.put("channel",channel);
cData.put("sender",sender);
cData.put("login",login);
cData.put("hostname",hostname);
cData.put("message",message);
cData.put("type","onMessage");
cData.put("cmdMap",cmdMap);
cData.put("args",l);
rMsg = cAct.action(cArgs, cData);
}
else { }
if(rMsg == null) { return; }
for(int i = 0; i < rMsg.size(); i++) {
this.sendMessage(channel,(String)rMsg.get(i));
}
}
//public void onConnect() {
//this.identify("111111");
//}
public static boolean makeFile(String name) {
boolean success = false;
try {
File file = new File(name);
success = file.createNewFile();
} catch (IOException e) {
e.printStackTrace();
}
return success;
}
public static boolean deleteFile(String name) throws IOException {
boolean success = (new File(name)). delete();
return success;
}
public static void write(BufferedWriter out, String line) throws IOException {
out.write(line);
out.newLine();
}
public boolean addCommand(String name, CommandAction cmd) {
cmdMap.put(name.toUpperCase(),cmd);
return true;
}
}
public class mXeBot {
public static void main(String[] args) {
final Bot bot = new Bot();
Scanner kbd = new Scanner(System.in);
System.out.print("Join what room on connect? ");
String room = kbd.nextLine();
bot.addCommand("roll", new CommandAction() {
public Vector action(String[] arg, HashMap cData) {
NumberFormat percent = NumberFormat.getPercentInstance();
int len = ((Integer)cData.get("args")).intValue();
String name = (String)cData.get("sender");
Vector ret = new Vector();
if(len==0) {
ret.add("Error: You didn't specify a dice amount. Try $help roll for help.");
} else {
String [] roll = arg[0].split("d");
int left = 0;
int right = 0;
try {
left = Integer.parseInt(roll[0]);
right = Integer.parseInt(roll[1]);
} catch (NumberFormatException e) {
ret.add("Error: Could not roll " + arg[0] + ". Try again, perhaps?");
return ret;
}
if(left > 30) {
ret.add("Error: You can't roll more than 30 of any type of die.");
return ret;
}
if(left < 0) {
ret.add("You can't roll less than none of something. That's basic math, and logic, " + name + ". What is wrong with you?");
return ret;
}
if(right < 0) {
ret.add("Pardon me, " + name + ", but I think it's a physical improbability for a die to have less than 0 sides.");
return ret;
}
if(right > 5000) {
ret.add("What are you trying to do, " + name + "? I mean, seriously. There's only so many physically feasible sides!");
return ret;
}
if(left == 0) {
ret.add("You can't roll a zero amount of something... That's like... crazy. Shame on you, " + name + ".");
return ret;
}
if(right == 0) {
ret.add("Well, isn't your sum obviously 0, " + name + "? How can something have 0 sides anyways?");
return ret;
}
Random rgen = new Random();
String buf = "";
double sum = 0;
int temp = 0;
for(int i = 0; i < left; i++) {
sum += (temp = rgen.nextInt(right)+1);
buf += (temp) + " ";
}
double total = left*right;
double per = sum/total;
ret.add(buf + "(Sum: " + (int)sum + " (out of a total possible " + (int)total + ") [" + percent.format(per) +"])");
}
return ret;
}
public String help () {
return "Usage: $roll <a>d<b>; rolls <a> <b>-sided dice.";
}
public boolean priv() {
return false;
}
});
bot.addCommand("nick", new CommandAction() {
public Vector action(String[] arg, HashMap cData) {
String type = (String)cData.get("type");
if(!type.equals("onPrivateMessage"))
return null;
int len = ((Integer)cData.get("args")).intValue();
String name = (String)cData.get("sender");
Vector ret = new Vector();
if(len==0) {
ret.add("Sorry, you seem to have forgotten to give a name.");
return ret;
}
String to = arg[0];
String admin = bot.getAdmin();
if(name.equalsIgnoreCase(admin)) {
bot.changeNick(to);
} else {
ret.add("I don't think you should be poking around so much.");
}
return ret;
}
public String help () {
return "Usage: $nick <nick>; changes nick to <nick>";
}
public boolean priv() {
return true;
}
});
bot.addCommand("join", new CommandAction() {
public Vector action(String[] arg, HashMap cData) {
String type = (String)cData.get("type");
if(!type.equals("onPrivateMessage"))
return null;
int len = ((Integer)cData.get("args")).intValue();
String name = (String)cData.get("sender");
Vector ret = new Vector();
if(len==0) {
ret.add("Sorry, you seem to have forgotten to give a channel name.");
return ret;
}
String to = arg[0];
String admin = bot.getAdmin();
if(name.equalsIgnoreCase(admin)) {
if(bot.inChan(to)) {
ret.add("Sorry, I'm already in that channel...");
return ret;`
}
bot.join(to);
} else {
ret.add("I don't think you should be poking around so much.");
}
return ret;
}
public String help () {
return "Usage: $join <chan>; joins <chan>";
}
public boolean priv() {
return true;
}
});
bot.addCommand("depart", new CommandAction() {
public Vector action(String[] arg, HashMap cData) {
String type = (String)cData.get("type");
if(!type.equals("onPrivateMessage"))
return null;
int len = ((Integer)cData.get("args")).intValue();
String name = (String)cData.get("sender");
Vector ret = new Vector();
if(len==0) {
ret.add("Sorry, you seem to have forgotten to give a channel name..");
return ret;
}
String to = arg[0];
String reason = "";
if(len==2) {
reason = arg[1];
}
String admin = bot.getAdmin();
if(name.equalsIgnoreCase(admin)) {
if(!bot.inChan(to)) {
ret.add("Sorry, I'm not in that channel...");
return ret;
}
bot.part(to,((reason=="")?bot.getReason():reason));
} else {
ret.add("I don't think you should be poking around so much.");
}
return ret;
}
public String help () {
return "Usage: $depart <chan> [reason]; departs from <chan>, optionally with [reason] as the reason.";
}
public boolean priv() {
return true;
}
});
bot.addCommand("hi", new CommandAction() {
public Vector action(String[] arg, HashMap cData) {
Vector ret = new Vector();
ret.add("Hello, "+((String)cData.get("sender"))+".");
return ret;
}
public String help() {
return "Usage: $hi; No real point.";
}
public boolean priv() {
return false;
}
});
bot.addCommand("help", new CommandAction() {
public Vector action(String[] arg, HashMap cData) {
Vector ret = new Vector();
Map cmdMap = (Map)cData.get("cmdMap");
String buf = "";
int length = ((Integer)cData.get("args")).intValue();
if(length==0) {
ret.add("AVAILABLE COMMANDS\n");
Set mapset = cmdMap.entrySet();
Iterator it = mapset.iterator();
while(it.hasNext()) {
Map.Entry ent = (Map.Entry)it.next();
String key = (String)ent.getKey();
if(!((CommandAction)ent.getValue()).priv())
buf += key.toLowerCase() + " ";
}
ret.add(buf);
ret.add("To get help for a specific command, type $help <cmd>");
} else {
String n = arg[0];
CommandAction cmd = (CommandAction)cmdMap.get(n.toUpperCase());
if(cmd.priv()) {
if(((String)cData.get("sender")).equals(bot.getAdmin()) && ((String)cData.get("type")).equals("onPrivateMessage")) {
bot.sendMessage(bot.getAdmin(),cmd.help());
return null;
}
}
if(cmd == null) {
ret.add("Error: command " + n + " not found.");
} else {
ret.add(cmd.help());
}
}
return ret;
}
public String help() {
return "Usage: $help [cmd]; gets help for cmd if given, else lists commands.";
}
public boolean priv() {
return false;
}
});
bot.addCommand("bash", new CommandAction() {
public Vector action(String[] arg, HashMap cData) {
Vector ret = new Vector();
int len = ((Integer)cData.get("args")).intValue();
String out ="";
if(len==0) {
out = fetch(-1);
} else {
int ind = -1;
try {
ind = Integer.parseInt(arg[0]);
} catch (NumberFormatException e) {
ret.add("Error: I expected a number... why not give me one?");
return ret;
}
out = fetch(ind);
}
String[] splitStr = out.split("\n");
for(int i = 0; i < splitStr.length; i++) {
String line = splitStr[i];
line = " " + line.replace("<br />","");
ret.add(line);
}
return ret;
}
private String getPage(int index) {
String url = "http://bash.org/?";
StringBuffer buf = new StringBuffer();
if(index==-1)
url += "random";
else
url += index;
try {
URL u = new URL(url);
InputStream in = u.openStream();
BufferedInputStream read = new BufferedInputStream(in);
int dat = 0;
while((dat = read.read())!=-1) {
buf.append((char)dat);
}
} catch (Exception e) {
return "Could not get the quote.";
}
return buf.toString();
}
private String fetch(int index) {
String page = getPage(index);
String ret = "";
String id = (new Integer(index)).toString();
if(page.matches("does not exist"))
ret = "Error: quote #"+index+" does not exist.";
else {
Pattern pat = Pattern.compile("<b>#([0-9]+)</b>",Pattern.MULTILINE | Pattern.DOTALL);
Matcher mat = pat.matcher(page);
if(mat.find()) {
id = mat.group(1);
}
pat = Pattern.compile("<p class=\"qt\">(.*?)</p>",Pattern.MULTILINE | Pattern.DOTALL );
mat = pat.matcher(page);
if(mat.find()) {
ret = "ID: " + id + "<br />\n"+mat.group(1);
} else {
ret = "Couldn't find quote with ID: " + id;
}
ret = ret.replace(""","\"");
ret = ret.replace("&","&");
ret = ret.replace("<","<");
ret = ret.replace(">",">");
ret = ret.replace(" ","&");
}
return ret;
}
public String help() {
return "Usage: $bash [num]; if num is given, fetches that quote from bash.org, else, gets a random quote.";
}
public boolean priv() {
return false;
}
});
bot.addCommand("op", new CommandAction() {
public Vector action(String[] arg, HashMap cData) {
Vector ret = new Vector();
String ch = (String)cData.get("channel");
if(bot.isValid(((String)cData.get("sender")))) {
ret.add("Sorry?");
return ret;
}
if(!bot.isOp(ch)) {
ret.add("Sorry, I don't have operator or higher privileges.");
return ret;
}
String name = "";
int len = ((Integer)cData.get("args")).intValue();
if(((String)(cData.get("type"))).equals("onPrivateMessage")) {
if(len != 2) {}
else {
ch = arg[0];
name = arg[1];
}
} else {
if(len == 0) {
return ret;
}
name = arg[0];
System.out.println(bot.isOp(ch) + "LOLZ?????");
}
bot.op(ch,name);
return ret;
}
public String help() {
return "Usage: $op [chan] <name>; op's name. (on chan if in pm)";
}
public boolean priv() {
return true;
}
});
bot.addCommand("dop", new CommandAction() {
public Vector action(String[] arg, HashMap cData) {
String ch = (String)cData.get("channel");
Vector ret = new Vector();
String name = "";
if(bot.isValid(((String)cData.get("sender")))) {
ret.add("Sorry?");
return ret;
}
if(!bot.isOp(ch)) {
ret.add("Sorry, I don't have operator or higher privileges.");
return ret;
}
int len = ((Integer)cData.get("args")).intValue();
if(((String)(cData.get("type"))).equals("onPrivateMessage")) {
if(len != 2) {}
else {
ch = arg[0];
name = arg[1];
}
} else {
if(len == 0) {
return ret;
}
name = arg[0];
}
if(name.equals(bot.getNick())) {
ret.add("I don't think so.");
}
System.out.println(bot.isOp(ch) + "LOLZ?????");
if(bot.isValid(((String)cData.get("sender")))) {
bot.deOp(ch,name);
}
return ret;
}
public String help() {
return "Usage: $dop [chan] <name>; deOp's name. (use chan if in pm)";
}
public boolean priv() {
return true;
}
});
//8ball./w
bot.connect();
bot.join(room);
}
}
Comments
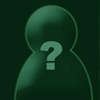