Welcome to HBH! If you have tried to register and didn't get a verification email, please using the following link to resend the verification email.
Fibonacci counter - C# Code Bank
Fibonacci counter
A program to count the Fibonacci sequence
//Be aware it could overflow for large iterations.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace FibonnacciCounter
{
class Program
{
static void Main(string[] args)
{
//Variable initialisation
string userInt;
int maxNumber = 0;
bool correctValue = false;
//so that only numbers get through
do
{
//User input
Console.Write(\"what number do you want to count up to >\");
userInt = Console.ReadLine();
try
{
maxNumber = int.Parse(userInt);
correctValue = true;
}
catch
{
Console.WriteLine(\"Not a valid number\");
}
} while (correctValue == false);
//More variable initialisation
int firstNumber = 0;
int secondNumber = 1;
int total = 0;
//Main iteration loop
for (int i = 0; i <= maxNumber; i++)
{
int temp = firstNumber;
firstNumber = secondNumber;
secondNumber = temp + secondNumber;
Console.WriteLine(\"{0} iteration is - {1}\", i, secondNumber);
//Checking if deviding by two leaves a remainder, if so its not part of the sequence
if (secondNumber % 2 == 0)
{
total += secondNumber;
}
}
Console.ReadLine();
}
}
}
Comments
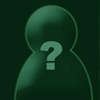
caseWest 12 years ago
The unndeeded backslashes are due to a problem with the inbuilt text editor, remove these (before and after the speech marks) for the code to work.