Input box in C#(looks like the vb one) - C# Code Bank
///Calling the inputbox:
/// <summary>
/// Replacement for VB InputBox, returns user input string.
///
///
///
///
/// <returns>string
public static string InputBox(string prompt,
string title, string defaultValue)
{
InputBoxDialog ib = new InputBoxDialog();
ib.FormPrompt = prompt;
ib.FormCaption = title;
ib.DefaultValue = defaultValue;
ib.ShowDialog();
string s = ib.InputResponse;
ib.Close();
return s;
} // method: InputBox
///Inputbox code in C#(this is a bit long)
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
namespace DocuTrackProSE
{
/// <summary>
/// Summary description for InputBox.
///
public class InputBoxDialog : System.Windows.Forms.Form
{
#region Windows Contols and Constructor
private System.Windows.Forms.Label lblPrompt;
private System.Windows.Forms.Button btnOK;
private System.Windows.Forms.Button button1;
private System.Windows.Forms.TextBox txtInput;
/// <summary>
/// Required designer variable.
///
private System.ComponentModel.Container components = null;
public InputBoxDialog()
{
//
// Required for Windows Form Designer support
//
InitializeComponent();
//
// TODO: Add any constructor code after InitializeComponent call
//
}
#endregion
#region Dispose
/// <summary>
/// Clean up any resources being used.
///
protected override void Dispose( bool disposing )
{
if( disposing )
{
if(components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
#endregion
#region Windows Form Designer generated code
/// <summary>
/// Required method for Designer support - do not modify
/// the contents of this method with the code editor.
///
private void InitializeComponent()
{
this.lblPrompt = new System.Windows.Forms.Label();
this.btnOK = new System.Windows.Forms.Button();
this.button1 = new System.Windows.Forms.Button();
this.txtInput = new System.Windows.Forms.TextBox();
this.SuspendLayout();
//
// lblPrompt
//
this.lblPrompt.Anchor =
((System.Windows.Forms.AnchorStyles)
((((System.Windows.Forms.AnchorStyles.Top |
System.Windows.Forms.AnchorStyles.Bottom)
| System.Windows.Forms.AnchorStyles.Left)
| System.Windows.Forms.AnchorStyles.Right)));
this.lblPrompt.BackColor = System.Drawing.SystemColors.Control;
this.lblPrompt.Font =
new System.Drawing.Font("Microsoft Sans Serif", 9.75F,
System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point,
((System.Byte)(0)));
this.lblPrompt.Location = new System.Drawing.Point(12, 9);
this.lblPrompt.Name = "lblPrompt";
this.lblPrompt.Size = new System.Drawing.Size(302, 82);
this.lblPrompt.TabIndex = 3;
//
// btnOK
//
this.btnOK.DialogResult = System.Windows.Forms.DialogResult.OK;
this.btnOK.FlatStyle = System.Windows.Forms.FlatStyle.Popup;
this.btnOK.Location = new System.Drawing.Point(326, 24);
this.btnOK.Name = "btnOK";
this.btnOK.Size = new System.Drawing.Size(64, 24);
this.btnOK.TabIndex = 1;
this.btnOK.Text = "&OK";
this.btnOK.Click += new System.EventHandler(this.btnOK_Click);
//
// button1
//
this.button1.DialogResult = System.Windows.Forms.DialogResult.Cancel;
this.button1.FlatStyle = System.Windows.Forms.FlatStyle.Popup;
this.button1.Location = new System.Drawing.Point(326, 56);
this.button1.Name = "button1";
this.button1.Size = new System.Drawing.Size(64, 24);
this.button1.TabIndex = 2;
this.button1.Text = "&Cancel";
this.button1.Click += new System.EventHandler(this.button1_Click);
//
// txtInput
//
this.txtInput.Location = new System.Drawing.Point(8, 100);
this.txtInput.Name = "txtInput";
this.txtInput.Size = new System.Drawing.Size(379, 20);
this.txtInput.TabIndex = 0;
this.txtInput.Text = "";
//
// InputBoxDialog
//
this.AutoScaleBaseSize = new System.Drawing.Size(5, 13);
this.ClientSize = new System.Drawing.Size(398, 128);
this.Controls.Add(this.txtInput);
this.Controls.Add(this.button1);
this.Controls.Add(this.btnOK);
this.Controls.Add(this.lblPrompt);
this.FormBorderStyle = System.Windows.Forms.FormBorderStyle.FixedDialog;
this.MaximizeBox = false;
this.MinimizeBox = false;
this.Name = "InputBoxDialog";
this.StartPosition = System.Windows.Forms.FormStartPosition.CenterScreen;
this.Text = "InputBox";
this.Load += new System.EventHandler(this.InputBox_Load);
this.ResumeLayout(false);
}
#endregion
#region Private Variables
string formCaption = string.Empty;
string formPrompt = string.Empty;
string inputResponse = string.Empty;
string defaultValue = string.Empty;
#endregion
#region Public Properties
public string FormCaption
{
get{return formCaption;}
set{formCaption = value;}
} // property FormCaption
public string FormPrompt
{
get{return formPrompt;}
set{formPrompt = value;}
} // property FormPrompt
public string InputResponse
{
get{return inputResponse;}
set{inputResponse = value;}
} // property InputResponse
public string DefaultValue
{
get{return defaultValue;}
set{defaultValue = value;}
} // property DefaultValue
#endregion
#region Form and Control Events
private void InputBox_Load(object sender, System.EventArgs e)
{
this.txtInput.Text=defaultValue;
this.lblPrompt.Text=formPrompt;
this.Text=formCaption;
this.txtInput.SelectionStart=0;
this.txtInput.SelectionLength=this.txtInput.Text.Length;
this.txtInput.Focus();
}
private void btnOK_Click(object sender, System.EventArgs e)
{
InputResponse = this.txtInput.Text;
this.Close();
}
private void button1_Click(object sender, System.EventArgs e)
{
this.Close();
}
#endregion
}
}
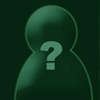
ghost 16 years ago
This is not coded by me, its coded by Les Smith from knowdotnet.com i just submitted this because its really awesome
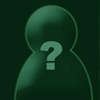
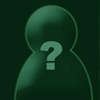
ghost 16 years ago
lol dragging and dropping isnt programming, its pure crap anyway, as far as i know, Visual C# has no drag-drop stuff, only Visual VB, which sucks coz it ruins the programming Drag and drop "programmers" has no knowledge in computers! they are just too lazy to actually learn and do original stuff!
thats why vb users are so spoiled..and silly sometimes..
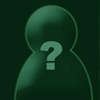
ghost 16 years ago
Ummm all of the Microsoft Visual products have drag n drop widgets…and they're actually badass nowadays with .NET Windows Forms. It's a bit lazy, but if you're just trying to make a quick decent looking Windows app sometime its definitely the way to go…you can actually focus on the actual logic instead of having to write all of that garbage. Also, FYI, the code from above is automatically generated word for word with the Windows Forms controls, which you can drag and drop. So its not crap. Yes it's better for noobs, but its really designed for RAD, rapid development, and to keep from writing a bunch of boilerplate. Don't hate on something unless you've tried it, and it has nothing to do with VB, thats the only thing I agree with you on and that is that VB sucks. I've seen some badass programs with GUI's made from the C# .NET Windows Forms..even made a few myself…you should check it out. Later.
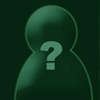
ghost 16 years ago
And if you want to write all that just for a damn textbox, then be my guest. But he's using everything from Windows Forms, which you can generate with a click of the mouse. Seems a bit ridiculous to me, but to each his own.
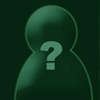
ellipsis 15 years ago
dragging and dropping is just a user friendly feature..why ridicule?
this source was coded in a drag and drop environment. so says the IDE generated code.
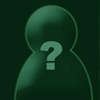
daiiani 14 years ago
Lol SpiderDude.
Do you manually dump Blind SQL injection dumps, just cuz using tools isnt hacking?
Thats dumb.
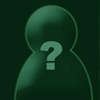
ghost 14 years ago
First of all, a large part of this WAS generated by the drag-and-drop function of visual studio's tools. Do you guys not see that it was generated code (as ellipsis says)?
Secondly, there are some severe inefficiencies with this code. Half those anchors aren't required, those "get" and "set" methods don't need to be explicitly created, anonymous functions would have saved the world here, ShowDialog is NOT the function that should be called (it works, but it is significantly inefficient as opposed to alternatives), and this program does not block correctly.
Someone needs to actually submit good C# programs to this website sometime. Maybe I will get around to doing it sometime…