Mapping Memory
Mapping Memory
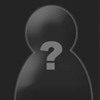
- 0x3C- Player X- float0x40- Player Y- float0x44- Player Z- float
- struct example{union{int something; // 0x4int somethingelse; // 0x4};};
- You get it? It all adds up.
- Because I do not feel like using powf, I will just use x * x and y * y and z * z. Since x * x is the same as x ^ 2.
- And so on.
Requirements: Basic knowledge of offsets, pointers, and structs.
In my last article a ‘safer’ way to inject DLL(s), I mentioned that it is possible to read data like so obj1>obj2>obj3->value from inside a DLL.
I have had quite a lot questions about this through PM lately. Therefore, I decided to write a quick walkthrough on mapping memory.
==================================
Lets assume we are writing a bot for a MMO and we want to calculate the distance between the player and the mob.
We have all the offsets and pointers that we need to do the trick.
========= POINTERS ========= BASE_ADDRESS: 0x00AF7464
NPC - [BASE_ADDRESS+0x1C]+0x24]+0x50+0*4]
PLAYER - [BASE_ADDRESS+0x34]
========= OFFSETS ========= Offset Name Type
0x3C- Mob X- float 0x40- Mob Y- float 0x44- Mob Z- float
0x3C- Player X- float 0x40- Player Y- float 0x44- Player Z- float
From inside a DLL we can read everything as you would in Address Space, I am going to give a quick demonstration on how structs work Behind The Scenes for those who do not know. (This includes some very basic ASM).
Lets say this would be the struct the game uses:
struct Player { int id; float x; float y; float z; };
An integer and float is 4 bytes. The struct always starts at 0x4.
ID will start at 0x4 and end at 0x8 Player X will start at 0x8 and end at 0xC Player Y will start at 0xC and end at 0x10 Player Z will start at 0x10 and end at 0x14
The value of where it starts is the offset. If two values have the same offset, add an union like so:
struct example { union { int something; // 0x4 int somethingelse; // 0x4 }; };
Hopefully you realize by now, that you can clone these structs as they point to a location. Which is exactly what we are going to do.
The first struct is what we call the CoreStruct, this will contain a pointer to a miscObj and a playerObj.
struct coreStruct { BYTE _1[0x1C]; miscObj *misc; BYTE _2[0x14]; playerObj *player; };
You might be wondering now, what is BYTE _1 and BYTE _2? This is what we call unknowns, we do not know what is between the two objects therefore we call everything between it BYTE _x[size].
So, BYTE _1[0x1C] is pointing to everything between 4 and 28 in decimal. The pointer misc is pointing to our next object the size of that is 4 so it will end at 32 in decimal. BYTE _2[0x14] is pointing to everything between 32 and 52. The pointer player is pointing to our next object the size of that is 4 so it will end at 56 in decimal.
You get it? It all adds up.
Now for training purposes work these structs out, you should have enough awareness to do so now. It will use the offsets and pointers I provided in the start.
struct infoObj { BYTE _1[0x3C]; // 60 float x; // 64 float y; // 68 float z; // 78 };
struct indexObj { infoObj *info; // 4 };
struct npcObj { BYTE _1[0x50]; // 80 indexObj *index; // 84 };
struct miscObj { BYTE _1[0x24]; // 36 npcObj *npc; // 40 };
struct playerObj { BYTE _1[0x3C]; // 60 float x; // 64 float y; // 68 float z; // 72 };
struct coreStruct { BYTE _1[0x1C]; // 28 miscObj *misc; // 32 BYTE _2[0x14]; // 52 playerObj *player; // 56 };
==================================
We can now start calculating the distance. You probably familiar with the Pythagorean theorem, it is kind of like that.
What we will do is the following:
x = Mob X - Player X y = Mob Y - Player Y z = Mob Z - Player Z
distance = squareroot(x ^ 2 + y ^ 2 + z ^ 2)
Because I do not feel like using powf, I will just use x * x and y * y and z * z. Since x * x is the same as x ^ 2.
At MMOs every resource, npc, player is assigned an index on the world map like so.
======= EXAMPLE ======= 1- Nothing 2- Nothing 3- Player 1 4- Nothing 5- Mob 1 6- Player 2 7- Mob 2 8- Nothing
And so on.
Knowing all this we can finally write our code.
float GetDistance(int ind) { coreStruct base = (coreStruct)0x00AF7464;
float x = (*base)->misc->npc->index[ind].info->x - (*base)->player->x;
float y = (*base)->misc->npc->index[ind].info->y - (*base)->player->y;
float z = (*base)->misc->npc->index[ind].info->z - (*base)->player->z;
float ground = sqrt(x * x + y * y);
return sqrt(ground * ground + z * z); // Total distance
}
Note that the index object is an array, thus index[0] will be 0x4, index[1] will be 0x8 and so on.
In this case, the index size is 0 to 768. So you would make a for loop and call GetDistance(i) to get the distance of every mob.
Hope this helped, you can always PM me. Goodluck.
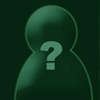
jackson600 13 years ago
:ninja::ninja::ninja::xx::radio::whoa::vamp::vamp::vamp::vamp::vamp::right::evil::evil::evil::evil::matey::love::matey::vamp::vamp::wow::radio::|;);):|:(:(:o:o:pB):D:D:@:angry::ninja::xx::whoa::vamp::love::matey:
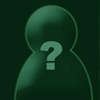
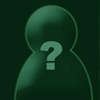
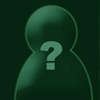