Advanced DLL Injection C/C++
Advanced DLL Injection C/C++
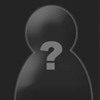
For those who are not familiar with DLL injection.
DLL injection is a method used to manipulate/execute code inside an other process. We can do this by ‘forcing’ the process to load a dynamic-link library (DLL), from then on we can run the code inside the process address space.
In this tutorial I will show you a safer way to inject a DLL.
- Why would you need a ‘safer’ way? Well, lets assume you’re writing a hack/bot for a game it will be somewhat inconvinient to get banned the entire time.
There’s obviously some other reasons, but i’m pretty sure you can think whether you need a ‘safer’ way or not for yourself.
I will try to slow it down for people who are not familiar with this topic, and I might even write some more basic ‘tutorials’ if people are interested. However, it would be useful if you know some ASM/C before you continue reading this tutorial.
–
- Why DLL injection instead of Code Injection? First of all, I won’t give you all the reasons since then we’ll still be here tomorrow. But if you inject a DLL you won’t have to allocate additional memory to read structures, instead you just point to the original data and read it out.
- More reasons below
- But doesn’t DLL(s) take up a few KBs in memory, thus more detectable? Well, depending on how much data you need and read you might be allocating just as much memory, if not more, against a very noticable loss in performance.
- Not to mention, that using DLL injection, we can read data like so obj1>obj2>obj3->value this is only a level3 pointer (ptr), imagine having a level5 one and see how many lines that would take using ReadProcessMemory().
- Back to detectability DLL injections USUALLY leaves trace of evidence, even if you try to hide it, it still leaves footprints behind. Whereas Code injection leaves no trace whatsoever.
–
- So what is the difference between the type of DLL injection you will be explaining and others? Normal DLL injection(s) exploit the Windows API to ‘force’ the process to load the DLL. This one won’t, this is probably one of the least detectable ways around.
–
The concept of what we will be doing is the following.
We will allocate memory so we can inject a stub that will make the process load our DLL. The advantage of this is that we can free the allocated memory later on whereas in methods such CreateRemoteThread you cannot.
Our stub. _asm { push 0xAAAAAAAA; pushfd; pushad; push 0xAAAAAAAA; mov eax, 0xAAAAAAAA; call eax; popad; popfd; ret; }
- What does this code do? It creates a placeholder for the return address, it then saves the flags and registers, then it creates another placeholder for the string address and LoadLibrary, then it calls LoadLibrary with the string parameter, restores the registers and flags, and return the control to the hijacked thread.
For more information about LoadLibrary check out: http://msdn.microsoft.com/en-us/library/ms684175(v=vs.85).aspx
- What is 0xAAAAAAAA? We don’t know the address 0xAAAAAAAA beforehand, therefore we will patch it on runtime.
Now we will have to allocate memory, to do this, we need to set some privileges. For our DLL name string we will need READ and WRITE privileges, for our stub we will need READ, WRITE and EXECUTE.
We will also have to open a handle to the process using the VM_OPERATION privilege.
If you’re unsure which functions you need take a look at: http://msdn.microsoft.com/en-us/library/ms684320(v=vs.85).aspx (OpenProcess) http://msdn.microsoft.com/en-us/library/aa366890(v=vs.85).aspx (VirtualAllocEx) http://msdn.microsoft.com/en-us/library/ms681674(v=vs.85).aspx (WriteProcessMemory)
For the next step we will have to suspend our thread. We will need to be able to GET and SET CONTEXT, SUSPEND the thread and also RESUME the thread using OpenThread.
http://msdn.microsoft.com/en-us/library/ms684335(v=vs.85).aspx (OpenThread)
-
Why do we have to suspend the thread? Well, we need to get the threads context which is the current state of all its registers amongst other information. We are just using it for the EIP register.
-
What is the EIP register? The EIP register contains the next instruction to be executed.
We will use the function GetThreadContext to grab the value of the next instruction to be executed. http://msdn.microsoft.com/en-us/library/ms679362(v=vs.85).aspx (GetThreadContext)
We now know where 0xAAAAAAAA should return to. Now all we have to do is patch the stub to have the proper pointers and force the thread to execute it, then we can resume the thread.
Now we can destroy the evidence, tee-hee. We will simply free the allocated memory, and close the handles.
http://msdn.microsoft.com/en-us/library/aa366894(v=vs.85).aspx (VirtualFreeEx)
And there goes the evidence.
–
Hope this convinced you that Code Injection is really not the only way to leave no evidence behind. For any questions you can always send me a PM.
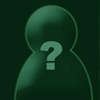
techb 14 years ago
This was a pretty good guide. I have a book called, "Greyhat Python" that goes over DLL, and code injection with python that is along the same lines as this article. If I get around to it, I'll post a write-up in parallel with this using python.
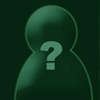
ghost 14 years ago
Well, this tutorial kind of teaches you to do Code injection too. Since you inject code that tells the target application to load the DLL. Most DLL injections are completely different though. The most common method is probably CreateRemoteThread.
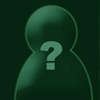
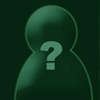
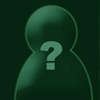
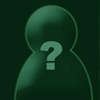
Night_Stalker 13 years ago
I know you're gone, but nice article, MolesteD_. I gotta agree with isarock and ellipsis. :happy:
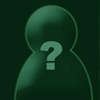