Anatomy of a Simple IP logger (PHP)
Anatomy of a Simple IP logger (PHP)
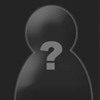
Anatomy of an IP logger (PHP)
In this little article, I’ll show you how to get someone’s ip by making them view an image on a website, so you provide them with a link like ‘yoursite.com/cool.jpg’, and their ip address shall be recorded to a text file on the server. The logger will be coded in PHP. PHP is a very versatile server side scripting language that can do just about anything. If you want to use PHP on your pc, just install Wamp (google it), it’s easier than installing all the components separately.
This concept I am about to show revolves around naming a folder on the web server something.jpg, giving it an extension so people think it is only an image, and then we can add an index.php file into the folder. I did not come up with this idea myself, but heard it form someone else, forgot who though. The actual code I am about to show was cobbled together by me though :)
Now, without further ado, the code:
// Ip recorder, use in the format yoursite.com/picture.jpg/index.php
// by wolfrat/deadata
//this picture variable defines which image to show the victim
$picture = "http://img.infotropic.com/w/w060529_2.jpg";
//this line just gets the visitor's ip address and stores it in the variable '$ip'
$ip = $_SERVER['REMOTE_ADDR'];
//this line tells the logger the name you want to use for the log file.
// if the logfile doesn't already exist, it will be created automagically
$logs = "logs.txt";
//here, we open the logfile for writing to. The 'a' means when we write
// data to the text file, it will be appended to the end of the file
$fh = fopen($logs, 'a') or die(" ");
//here we put the ip address into a variable called 'string data'
// the backslash-n starts a new line in the text file, to make it easier to read
$stringData = "$ip \n";
//here we write to the text file what is in the stringdata variable
fwrite($fh, $stringData);
//now we close the file
fclose($fh);
//and finally we echo some html back to the user's browser so they see an image
echo "<img src='$picture'>";
?>```
Implementation:
Put something like the code above into a text file and call it index.php, upload to a web server that has support for php, and place it in a folder with a name like amazing_pic_of_amy_lee.jpg
Thanks for reading my article. I hope it helps.
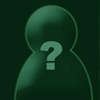
ghost 17 years ago
fucking stupid, and overcomplicated. just put the REMOTE_ADDR line on any page of the site, you don't need to do all this image shit.
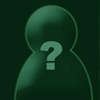
ghost 17 years ago
yeah, but people are more trusting of what looks like a simple image file. Also this article is to teach n00bs about writing stuff to file in php, and a way to hide stuff, more than anything else.
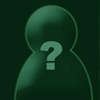
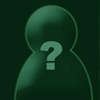
ghost 17 years ago
well some haven't i say very good it shows what each line of code is actually doing and gives an application for it ^_^
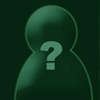
ghost 17 years ago
Yeah, i think its kinda cool :P, like if u want it reallly hidden, u could just make it a banner :P
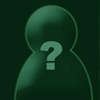
ghost 17 years ago
picture?hidden? :| let say this is your logger, logger.php :
$handling1 =fopen("logfile.txt","a+"); $step1 = "".$_SERVER['REMOTE_ADDR']."" ; $step2=addslashes(htmlentities("<br/>".$_SERVER['HTTP_USER_AGENT']))."<hr>"; $handling2 = fwrite($handling1,$step1); $handling3 = fwrite($handling1,$step2); fclose($handling1);
and now we edit your index page:
require_once "logger.php";
who can ever see their ips and useragents get logged? also,when you log useragents,be aware for XSS!!! then,to check for ip like that,is not very good? any proxy ip will validate as legitimate user ip's? but anyways,have fun with your php practices :happy:
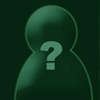
ghost 17 years ago
hmm… how about you use .htaccess to rewrite rules on the extension .png to read as php, then make a php file that logs the user ip then displays an image using the php image library? then you can use it on any site and they wont suspect a thing ^^
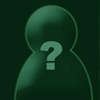
ghost 17 years ago
mr noob: he'd prolly have to use a database, then, instead of a text file.
but that's what I was going to suggest.
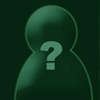
ghost 17 years ago
no need for a db… just use fopen and fwrite, then imagecreatefrompng and set the content type to png. easy lol
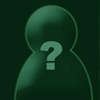
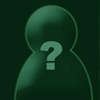
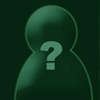
ghost 17 years ago
All you need is a PHP file written in a .gif, a real gif image, noob PHP skills, and 1 line in a .htaccess to allow .gif file types to handle PHP.
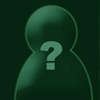
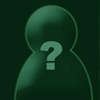
ghost 15 years ago
If you visit my profile and then go to http://myspecialsite.site90.net/ip.html you can see your ip.