Gaining elevated rights in a secure hosting environment
Gaining elevated rights in a secure hosting environment
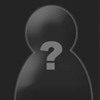
The code for this articles was inspired when I was infuriated by the fact the hosting company would not allow read/write access to my own web application’s hard drive. I was also restricted from using some of my favorite functions including creating server side activeX objects. Finally, I got fed up. I knew that I had rights to the file system on the server. I just need to write some code that would allow me to temporarily logon with elevated privileges from within my web application. So here’s the code. If you need help using or have any other question’s PM me.
Windows Identity Impersonation in Vb.Net
In this Article Summary How to use this code Identity Impersonate with VB.Net code How It Works.
Summary
This technical article reviews the process of creating Visual Basic.Net 2003 Shared class to perform Windows Identity Impersonation on a remote PC. This code must be ran on the remote PC to logon to that PC. You can use it on a local machine to temporarily allow a restricted users administrative rights on a PC, via WindowsIdentity Impersonation. I have used this code on remote web servers to allow The ASP.Net Account to operate with administrative rights to create activex objects and allow read/write system.io and create scripting.filesystemobject's internerexplorer.application object, etc, on restricted server where WindowsIndenty Impersonation is prohibited because the ASP.net account is restricted from creating login Tokens and do to low trust or restricted trust .net security configurations.
How to Use the Windows Identity Impersonation Code To use this code
Call the function main() to perform the Windows Identity Impersonation Login. Call the undoImpersonation method to log the use out.
Imports System Imports System.Runtime.InteropServices Imports System.Security.Principal Imports System.Security.Permissions Imports Microsoft.VisualBasic <Assembly: SecurityPermissionAttribute(SecurityAction.RequestMinimum, UnmanagedCode:=True), _ Assembly: PermissionSetAttribute(SecurityAction.RequestMinimum, Name:="FullTrust")> Module Module1
End Module Public Class impersonate '<System.Security.Permissions.PermissionSetAttributeSystem. Security.Permissions.SecurityAction.Demand File:="test.txt" Name:="FullTrust"(,),> _ ' Private Sub Page_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) ' 'Put user code to initialize the page here ' Call Main() ' 'Dim ie As Object = Server.CreateObject("internetexplorer.application") 'End Sub Private Declare Auto Function LogonUser Lib "advapi32.dll" (ByVal lpszUsername As [String], _ ByVal lpszDomain As [String], ByVal lpszPassword As [String], _ ByVal dwLogonType As Integer, ByVal dwLogonProvider As Integer, _ ByRef phToken As IntPtr) As Boolean
<DllImport("KERNEL32.DLL")> _ Public Shared Function FormatMessage(ByVal dwFlags As Integer, ByRef lpSource As IntPtr, _ ByVal dwMessageId As Integer, ByVal dwLanguageId As Integer, ByRef lpBuffer As [String], _ ByVal nSize As Integer, ByRef Arguments As IntPtr) As Integer
End Function
Public Declare Auto Function CloseHandle Lib "kernel32.dll" (ByVal handle As IntPtr) As Boolean
Public Declare Auto Function DuplicateToken Lib "advapi32.dll" (ByVal ExistingTokenHandle As IntPtr, _ ByVal SECURITY_IMPERSONATION_LEVEL As Integer, _ ByRef DuplicateTokenHandle As IntPtr) As Boolean
'GetErrorMessage formats and returns an error message 'corresponding to the input errorCode. Public Shared Function GetErrorMessage(ByVal errorCode As Integer) As String Dim FORMAT_MESSAGE_ALLOCATE_BUFFER As Integer = &H100 Dim FORMAT_MESSAGE_IGNORE_INSERTS As Integer = &H200 Dim FORMAT_MESSAGE_FROM_SYSTEM As Integer = &H1000
Dim messageSize As Integer = 255 Dim lpMsgBuf As String Dim dwFlags As Integer = _ FORMAT_MESSAGE_ALLOCATE_BUFFER Or FORMAT_MESSAGE_FROM_SYSTEM Or FORMAT_MESSAGE_IGNORE_INSERTS
Dim ptrlpSource As IntPtr = IntPtr.Zero Dim prtArguments As IntPtr = IntPtr.Zero
Dim retVal As Integer = FormatMessage(dwFlags, ptrlpSource, errorCode, 0, lpMsgBuf, _ messageSize, prtArguments) If 0 = retVal Then Throw New Exception("Failed to format message for " & _ "error code " + errorCode.ToString() + ". ") End If
Return lpMsgBuf End Function 'GetErrorMessage ' Test harness. ' If you incorporate this code into a DLL, be sure to demand FullTrust. <PermissionSetAttribute(SecurityAction.Demand, Name:="FullTrust")> _ Public Overloads Shared Function Main(byval username as string, byval password as string, _ byval machineName as string) As Object
Dim tokenHandle As New IntPtr(0) Dim dupeTokenHandle As New IntPtr(0) Try
Dim UserName, MachineName As String
' Get the user token for the specified user, machine, and password using the ' unmanaged LogonUser method. ' 'For a domain logon set the Machine name as the domain logon 'For a local login set the Machine name to nothing – ie, don't set the varialble 'MachineName = Environment.MachineName ''HttpContext.Current.Response.Write("Enter " & _ "the name of a machine on which to log on: " & MachineName)
space() UserName = strUserName ''HttpContext.Current.Response.Write("Enter the login of a user" & _ " on {0} that you wish to impersonate: on " & UserName) space()
'UserName = Console.ReadLine() Dim password As String = StrPassword ''HttpContext.Current.Response.Write("Enter the password for {0}: " & password) space() Const LOGON32_PROVIDER_DEFAULT As Integer = 0 'This parameter causes LogonUser to create a primary token. Const LOGON32_LOGON_INTERACTIVE As Integer = 2 Const SecurityImpersonation As Integer = 2
tokenHandle = IntPtr.Zero dupeTokenHandle = IntPtr.Zero
' Call LogonUser to obtain a handle to an access token. Dim returnValue As Boolean = LogonUser(UserName, _ MachineName, password, LOGON32_LOGON_INTERACTIVE, _ LOGON32_PROVIDER_DEFAULT, tokenHandle)
''HttpContext.Current.Response.Write("LogonUser called.") space() If False = returnValue Then Dim ret As Integer = Marshal.GetLastWin32Error() ''HttpContext.Current.Response.Write("LogonUser failed" & _ " with error code : {0} " & ret) space() '' HttpContext.Current.Response.Write(ControlChars.Cr & _ "Error: [{0}] {1}" + ControlChars.Cr, ret, GetErrorMessage(ret))
Return Nothing End If
Dim success As String If returnValue Then success = "Yes" Else success = "No" ''HttpContext.Current.Response.Write(("Did LogonUser succeed? " + success)) space() ''HttpContext.Current.Response.Write(("Value of Windows NT token: " & _ tokenHandle.ToString())) space() ' Check the identity. ''HttpContext.Current.Response.Write(("Before impersonation: " & _ WindowsIdentity.GetCurrent().Name)) space() Dim retVal As Boolean = DuplicateToken(tokenHandle, _ SecurityImpersonation, dupeTokenHandle) If False = retVal Then CloseHandle(tokenHandle) ''HttpContext.Current.Response.Write("Exception thrown " & _ "in trying to duplicate token.") space() Return Nothing End If
' TThe token that is passed to the following constructor must ' be a primary token in order to use it for impersonation. Dim newId As New WindowsIdentity(dupeTokenHandle) Dim impersonatedUser As WindowsImpersonationContext = newId.Impersonate()
' Check the identity. 'HttpContext.Current.Response.Write(("After impersonation: " & _ WindowsIdentity.GetCurrent().Name)) 'Dim objie = CreateObject("internetExplorer.application")
'Dim sr As New System.IO.StreamWriter(HttpContext.Current.Server.MapPath("/nexperts.org/test.text"))
'sr.WriteLine("hello") 'sr.Close()
'' Stop impersonating the user. 'space() 'impersonatedUser.Undo()
'' Check the identity. 'HttpContext.Current.Response.Write(("After Undo: " + WindowsIdentity.GetCurrent().Name)) 'space() '' Free the tokens. Dim obj(3) obj(0) = impersonatedUser obj(1) = tokenHandle obj(2) = dupeTokenHandle Return obj 'If Not System.IntPtr.op_Equality(tokenHandle, IntPtr.Zero) Then ' CloseHandle(tokenHandle) 'End If 'If Not System.IntPtr.op_Equality(dupeTokenHandle, IntPtr.Zero) Then ' CloseHandle(dupeTokenHandle) 'End If Catch ex As Exception HttpContext.Current.Response.Write(("Exception occurred. " + ex.Message)) End Try End Function 'Main 'Public Shared Sub undoimpersonate(ByVal token As System.Security.Principal.WindowsImpersonationContext, _ 'ByVal inptr As IntPtr, ByVal dupetokenhandle As System.IntPtr, ByVal tokenhandle As System.IntPtr) Public Shared Function undoimpersonate(ByVal obj) Dim token As System.Security.Principal.WindowsImpersonationContext = obj(0) token = obj(0) token.Undo() Dim tokenhandle As System.IntPtr = obj(1) Dim dupetokenhandle As System.IntPtr = obj(2)
If Not System.IntPtr.op_Equality(tokenhandle, IntPtr.Zero) Then CloseHandle(tokenhandle) End If If Not System.IntPtr.op_Equality(dupetokenhandle, IntPtr.Zero) Then CloseHandle(dupetokenhandle) End If End Function Public Shared Sub space() '' HttpContext.Current.Response.Write(" ") End Sub End Class
How It Works
How it works. The code imports system dll functions login a user, duplicate a user token, and close the handle for the token. The user is logged onto the remote system effectively performing pseudo windows identity impersonation. Any applications and processes from that point will run under the account of the logged in user, with the permissions of that account. Afterwards calling the undoimpersonation closes the windows identity impersonation by closing the handle on the duplicated windows tokens, and returns code execution back to the original process the code was executing under. For more tips and advice as to how you can use windowsIndentity Impersonation or the WindowsIdentity Impersonate method in your .Net Applications, PM me.
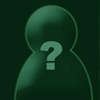
ghost 18 years ago
good work, dude. Point taken. But, " … the hosting company would not allow read/write access to my own web application’s hard drive" sounds a bit off. You did a great job however…
Oh, by the way, it's ELEVATED, not EVELATED. I still rate it good!! Congrats.
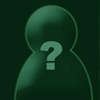
Neo_Chalchus 18 years ago
Thats pretty cool, saved to folder incase ever needed. You may want to reword some of it, certain bits are a little…confusing. Anyway, thats pretty good. You can probobly submit that to the code bank as well if you comment it. rated very good, for originality and not the same exploit over and over again
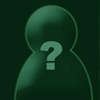
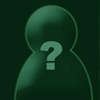
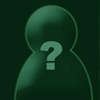