Javascript 16 *may contain spoilers*
Javascript 16 *may contain spoilers*
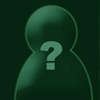
Part 1: Mathematical Breakdown
When you view the source code for the page, we see that whether or not you pass the challenge is based on a javascript function called “Check”. With a quick glance at the function, it is clear that it is taking your password input and converting it into a number using some algorithm. Once your string is converted, it is then compared to a number that they have hard coded into the html (88692589).
After determining this preliminary information, it is clear that we must understand how our string is being converted, so that we can craft a string appropriately. As you can tell, there is a loop that determines the value of the variable “sum”, which is the value of our converted string. In order to understand how the conversion works, we must understand this line of code:
sum = sum + (indexni)(indexi*i);
What is going on here? This loop is executing n times, and each time it executes a value determined by the formula (indexni)(indexi*i) is being added to the variable “sum”. Here’s where the mathematical analysis comes in. Because this is in a loop and it’s adding a value determined by a formula each time it iterates through the loop, this routine can also be expressed as a summation. Let’s take another look at the formula:
(indexni)(indexi*i)
Everything in this expression is separated by a multiplication operation, so the parenthesis and order that the terms are grouped in do not matter. Therefore, this can be rewritten like:
indexindexiii*n
This expression obviously can be simplified further:
(index^2)*(i^3)*n
Now we can put this into our summation format:
sum[(index^2)*(i^3)*n] from i=0 to n-1
The summation bounds are from i=0 to n-1 because the loop in the code goes from i=0 to i<n. Therefore the largest value that i may be is n-1. This summation can be simplified, however. In the context of the summation, n is going to be chosen to be some constant and can therefore be pulled out of the summation leaving us with the formula:
n*(sum[(index^2)*(i^3)] from i=0 to n-1)
The next thing to determine is what this sum is going to be equal to. Looking back at the original source code for the page, we can see that our variable “sum” is being compared to another variable “checksum,” which is chosen when the function is called. Therefore we must look at the function call to see what number “checksum” is equal to. The function call tells us that the number that our summation must equal is 88692589. The equation that has been built so far:
n*(sum[(index^2)*(i^3)] from i=0 to n-1) = 88692589
At first glance, it would appear that we have built an equation that will help us determine potential values of n, but we have missed a critical detail. Let’s look again at when the variable “sum” was declared:
var sum = 1;
The variable “sum” actually starts off at 1, and we have been assuming that it starts as 0, so we must adjust our equation accordingly:
1 + n*(sum[(index^2)*(i^3)] from i=0 to n-1) = 88692589
Now we can use some simple algebra to isolate our summation on one side of the equation:
(sum[(index^2)*(i^3)] from i=0 to n-1) = 88692588/n
At this point it may be unclear what this equation that we have gotten can determine for us, but let’s think about it logically. We know that “index” and “i” are both natural numbers. What do you get when you square some natural number, cube another natural number and then multiply them together? You get back another natural number. What do you get when you add together a bunch of natural numbers? You get a natural number. Therefore the sum on the left hand side of our equation must be some natural number because it is simply adding together the product of a bunch of natural numbers. This means that the right side of our equation must be a natural number as well, or in other words, n must divide 88692588 perfectly. It might still be unclear why this helps us because up until this point, we haven’t determined what n represents, so let’s look back at the code.
var n = entry.length;
Now we can see why knowing what n is can be very powerful. The variable n is the length of the string that we enter in (the password). The form will only let you enter in a maximum of 20 characters so our upper bound for n must be 20. Also, you can easily determine by trial and error that it is impossible to make a string under 10 characters that could be generate a number large enough to match the checksum. Therefore we must find a number for n between 10 and 20 that divides 88692588 perfectly. Using trial and error we can find that the only values of n that perfectly divide into 88692588 between 10 and 20 are 12 and 18. 18 seems like it would be a large length for a password, so odds are that the password is going to be of length 12.
Part 2: Brute Forcing the Password
Now that we know the length of the string, brute forcing the password is an easy task. Here’s an idea you can use that help you get a valid password in seconds. We know what characters are possible for the string, so we can build each string systematically using that list (the spaces at the beginning can be left out because the space has an index of 0 and won’t contribute to the sum at all).
Step 1 - convert the “Check(checksum)” method to the language of your choice Step 2 - build each string systematically i.e. string 1 = “aaaaaaaaaaaa”, string 2 = “aaaaaaaaaaaz”, string 3 = “aaaaaaaaaaae”, … , string n = “@@@@@@@@@@@@” Step 3 - check each string that you’ve built using the “Check(checksum)” method Step 4 - once you find a string that gives you a valid sum, enter the password on HBH and complete the challenge
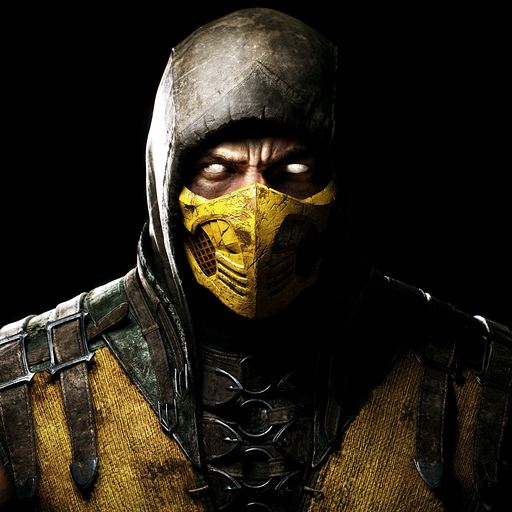
elmiguel 13 years ago
Nice analysis on the mathematics, but I have to point out that you are only half right on part two. Remember, clues on this challenge were given out in the forum. Using the brute force method is the way to complete this challenge but not in a way that one might think. This challenge was more of a riddle that made you create or own tool for education rather then practical use. Generating strings is not the method in this case as splitting common words with common number is. A lot of the material here has been explained before, but will give credit on the analysis and re-defining of the equation.
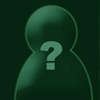
dopeboimag1k 13 years ago
Thanks for the comment. I was actually unaware that hints were given out in the forums. I didn't do a very good job on the second part so I'm glad your comment can add further clarification.:D
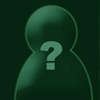
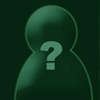
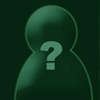
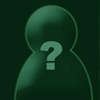
deepfreeze 8 years ago
As you pointed out in part 2: "Spaces have an index of 0" which is definitely true. In theory, the correct password can have any amount of spaces added anywhere in it and the basic16 script will still accept it. :o