Using Python for timed challenges.
Using Python for timed challenges.
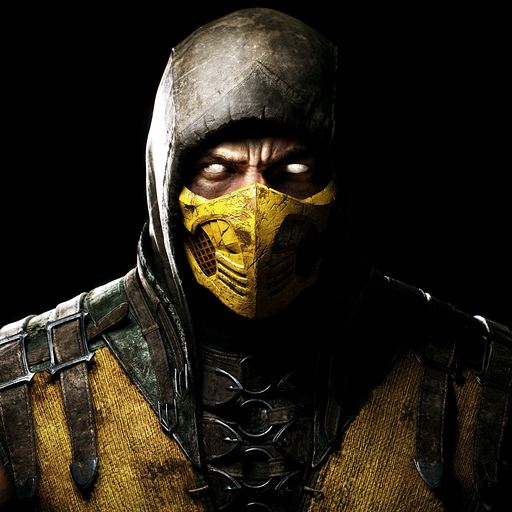
- Make a variable to hold our cookie headers.
- Make a variable to hold our URL address, this will make it easier to change.
- Make a variable to open a request to our destination
- Add the data key ‘Referrer’ with the host HBH
- Make a variable to open the site
- Make a variable to store our data for manipulation
- Make a POST variable to hold our answer, ‘ans’ is like having ?ans=answer and the end of the url
- Also make a note that you need to urlencode the values to be able to send the data(this is why we imported urllib).
Using Python for timed challenges.
Hello everyone. I thought I would make this tutorial to help those who wish to use Python for the timed challenges. Python is a very easy and useful language that can be used multiple applications. Today we will be using it to do http requests to receive data from and send data to the web page located on the Hellbound Hackers server.
I am assuming that you know the basics of python. If not, please visit the few articles here at HBH or look at the documentation off of the python website. Or Google “Python tutorial” that will give you enough to start off with. Also YouTube has some great learning videos as well.
http://docs.python.org/tutorial/
Anyway, enough with the formalities let�s get explainning shall we! To make http requests in python you will need to use some module libraries. We will use urllib, urllib2. We will also need to use the RegEx module: re. This module enables us to use regular expresblockedsions to search through the received data from the http request. Then we will copy the time() function from the time module. This is not necessary but will be helpful to know if your script is taking too long.
First we import our modules:
#Python 2.6.2
import urllib, urllib2, re from time import time
#Now that our modules are imported into our script we will set some variables to store our information request, time, etc.
t=time()
Make a variable to hold our cookie headers.
headers = {‘COOKIE’: ‘PHPSESSID=<session_id>; fusion_user:<id.hash>’}
Make a variable to hold our URL address, this will make it easier to change.
url = ‘http://www.hellboundhackers.org/challenges/timed//index.php’
Make a variable to open a request to our destination
req = urllib2(url, None, headers)
Add the data key ‘Referrer’ with the host HBH
req.add_header(‘Referrer’, ‘http://www.hellboundhackers.org/’)
Make a variable to open the site
response = urllib2.urlopen(req)
Make a variable to store our data for manipulation
page = str(response.read())
So, now if we do a print of our ‘page’ we can see the page data.
print page print time()-t
[page output] [time output]
Alright, we now have are data stored correctly. Now, we will use the ‘re’ module to search our data. I will only use examples here as I believe it is up to you to learn regular expresblockedsions.
page = re.match(’[regex here]’)
Tip: use your expresblockedsions inside a detailed search string::
word = re.findall(‘random string: (.*) and answer’, page)
This will find the specific search string we are looking for and return it to be stored. Also, note that I am using findall() this will store it in a list (array).
print page
[‘found string’]
You will need to use the index of the list (array) to able to use it correctly.
print page[0]
found string
Although that this not the best practice for using re.findall() to store the string. The best practice for you to use will be this:
word = page[page.find(’random string: ’)+15:page.find(‘and answer’)]
print word
found word
Note: page[page.find(’random string: ’)+15:page.find(‘and answer’)]. We are accessing the page variable at the section where are regex will be. But, there is a something I want to point out. the +15 after: page.find(’random string: ’). I am adding 15 chars to the beginning of the starting location. Otherwise, we would get:
print word
random string: [found string]
Ok, so now that we have the basics on getting and storing our data you just need to figure out what exactly you need to do for the challenge. This is really easy to do (except for Timed 7, easy to say then do).
Once you have the correct answer for the challenge you need to post it back to the page (or send it back at a certain url). This really simple since we created are req variable to make a request we can just call it again but with POST instead of the GET method.
answer = your solved answer for the challenge
Make a POST variable to hold our answer, ‘ans’ is like having ?ans=answer and the end of the url
Also make a note that you need to urlencode the values to be able to send the data(this is why we imported urllib).
post = urllib.urlencode({‘ans’: answer})
#requesting again with the new post data response = urllib2.urlopen(req, post)
Well that�s it! I hope this helps in your challenges and also to understand on how to make requests to and from a web site.
-elmiguel
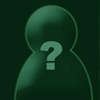
ynori7 15 years ago
I think this article would have been better if it was made more general. And RegEx is not required; I didn't use it for any of the timed challenges. Average.
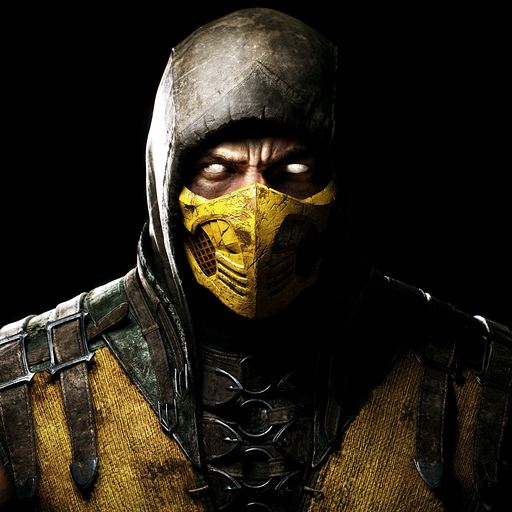
elmiguel 15 years ago
Thank you for your comment. As I am still learning all the possibilities that python has to offer, I realize that RegEx isn't required but at the moment it is more of habbit then anything.
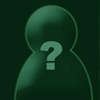
ghost 15 years ago
This is disgusting compared to Perl :angry: Nevertheless, pretty good, I plan on learning Python sometime.
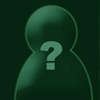
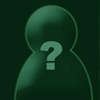
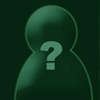
korg 15 years ago
Really not that bad, should help some people just starting in python use some basics.
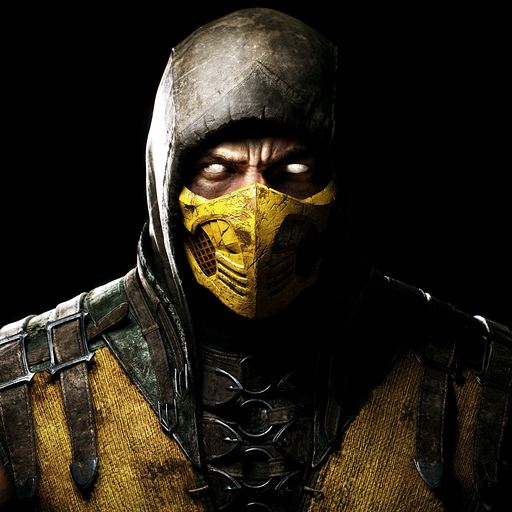
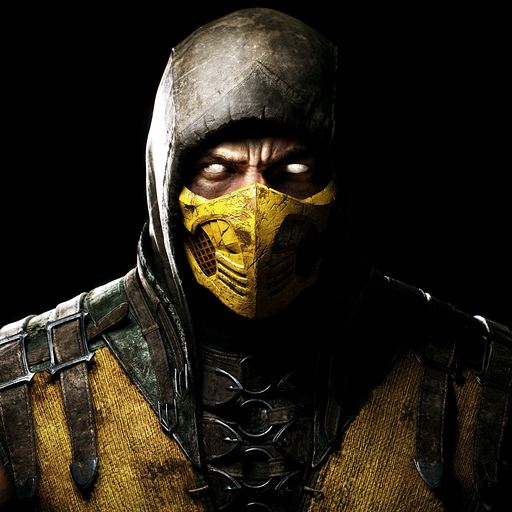
elmiguel 15 years ago
was aiming* not was am. And also, some, not dome. Holy crap that was a bad sentence.
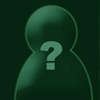
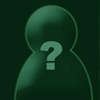
ADIGA 13 years ago
php curl seems to be more understandable to me :-S and i wount rate it because i have no idea how to rate it.