Timed Challenges 1,2,3,4,5,8 Tutorial
Timed Challenges 1,2,3,4,5,8 Tutorial
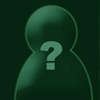
This tutorial is splitted into 8 chapters, 1 general chapter and 1 chapter for each challange. The first chapter, called “General” covers the basics you need for every Timed Programming Challange, so its a good idea to read it first, because I will not go into the deep of the basic stuff at the challenge chapters, except for Timed Challange 5. So if you are new to this Timed Challanges, you should start with Timed 5.
Table of Contents
0.) General 0.1) What we need for all Timed Challanges 0.2) The Main Problems 0.2.1.) The Time Problem 0.2.2.) Greasemonkey 0.2.2.1) The Greasemonkey-Header 0.2.2.2) The Greasemonkey-Body 0.2.3.) Getting the needed informations 0.2.3.1) The Match-Function 0.2.3.2) The Substr- and Substring-Functions 0.2.4.) Generate the answer 0.2.5.) Sending back the answer 0.2.5.1) Filling out a existing form 0.2.5.2) Submitting with GET-Method 0.2.5.3) Submitting with POST-Method
1.) Timed Challange 1 1.1) What we have to do 1.2.) How we do it
2.) Timed Challange 2 2.1) What we have to do 2.2.) How we do it
3.) Timed Challange 3 3.1) What we have to do 3.2.) How we do it
4.) Timed Challange 4 4.1) What we have to do 4.2.) How we do it
5.) Timed Challange 5 5.1) What we have to do 5.2.) How we do it 5.2.1.) Writing the Greasemonkey-script-header 5.2.2.) Programing the Javascript 5.2.2.1.) Getting the RANDOMNUMBER 5.2.2.2.) Calculating the ADDINGNUMBER 5.2.2.3.) Changing the sign to “plus_” or “minus_” 5.2.2.4.) Submiting the answer 5.3.) Tips
8.) Timed Challange 8 8.1) What we have to do 8.2.) How we do it
9.) Epilogue
##################################################
0.) General
All Timed Programming Challanges are working the same way, so I’m introducing the basics now.
0.1) What we need for all Timed Challanges
-medium knowledge of Javascript Documentation: http://www.w3schools.com/jsref/default.asp
-basic knowledge of HTML Documentation: http://www.w3.org/MarkUp/
-Greasemonkey extension for FF(or IE) Documentation: http://diveintogreasemonkey.org/toc/ Download: https://addons.mozilla.org/en-US/firefox/addon/748
-an javascript editor(or notepad, if you dont need syntaxhighlighting) Download: http://notepad-plus.sourceforge.net/uk/site.htm
-a not too slow computer with a fast internet connection
0.2) The Main Problems
In all Timed Challanges we have to solve the same problems, which are…
0.2.1.) The Time Problem
These challanges are called Timed Programming Challanges, because we only have some seconds between we open the challange page and send back our answer. To be in time we need a fast internet connection first, so we dont loose time while loading the challange page and sending back the answer. The second point is the time, our program needs to find out the answer. So we need a fast computer and a good and fast sourcecode. Also we don’t have the time, to copy words or numbers out of the website and paste them into our program, therefore we use a greasemonkey-script to solve these challanges. If you are out of time, try to close every not needed program, stop downloading/uploading and improve your code.
0.2.2.) Greasemonkey
Because we don’t have the time to do something manually, we have to code a program, which automates it and we do this with Greasemonkey. Greasemonkey is an extension for Firefox and Internet Explorer and is used to automate the manipulation of specific websites with greasemonkey-scripts. So we have to code a greasemonkey-script, which is automatically started, when we open the challange page and then it has to find out the answer and submit it. That sounds difficult, if you never have used it, but its very simple, if you know, how to code a javascript. A Greasemonkey-script consists of 2 locical parts. The first part is the header, which sets the script informations and rules. The second logical part is normal Javascript code, which makes the script do something(call it body, if you want). Save both together in a *.user.js file and drag it into your browser with installed greasemonkey extension and your script is active and ready to work, after you confirmed the popup window.
0.2.2.1) The Greasemonkey-Header
The first few lines of our greasemonkey-script is the header. In this example I used the header for Timed Challange 5. This should look like:
*** Note: I start the codelines with “XX: “, where “XX” is the number for the line, so dont use the linenumber information in your script! ***
01: // ==UserScript==
02: // @name
HBH Timed Challange 5
03: // @namespace
http://www.unimportantdomain.com/
04: // @description
This script does this and that
05: // @include
http://www.hellboundhackers.org/challenges/timed/timed5/*
06: // @exclude
http://www.hellboundhackers.org/challenges/timed/timed5/?
07: // ==/UserScript==
The “// ==UserScript==”, which has to be our first line in our script, starts the Greasemonkey-header(like the “” tag in a HTML-document). The “// ==/UserScript==” in the 7th line closes the Greasemonkey-header(like the “” in HTML).
Between them, we can specify the informations and rules for our Greasemonkey-script. They are build up like "// @PARAMETER VALUE"
, like “” in HTML.
In our case we have to specify a name for our script and we do that with "// @name HBH Timed 5"
in our 2nd line. In the third line we specify a path. Its unimportant, which path we choose, because the main function of this is, to make the script “unique”. If you have by accident 2 scripts with the same ‘name’, Greasemonkey will compare their ‘namespace’ and look, if it’s still the same. The 4th line specifies a description, what our script is doing.
But the most important thing here are the 5th and 6th lines. The 5th line lets the script run each time, we open a file in our specified path. The “*” is the wildcard symbol, which we use to run the script everytime, we open any file in “http://www.hellboundhackers.org/challenges/timed/timed5/”.
Because we don’t want a endless loop, when we send back our answer with GET-parameters, we use the 6th line which prevents, that the script would be started, if we open a file with parameters. So, the 6th line is similar to the 5th line but it don’t specify, when the script will run, instead it specifies when it should not run. If we want, that our script is (not) executed on different sites, we can add more "@include"
or "@exclude"
lines, but for Timed 1-5 and 8 we only need one line.
0.2.2.2) The Greasemonkey-Body
After we closed the Greasemonkey-header with “// ==/UserScript==”, we can start to code our Greasemonkey-body with javascript. We dont have to open or close the body, we just start to code. Beside javascript, we could use special greasemonkey-function, but we only need them for Timed 6. So just code like we would code a javascript which is located between “” and “” HTML-tags on the challange page.
0.2.3.) Getting the needed informations
Our goal is to send back a answer to the challange page, but before we can do that, we have to find out the informations, we need, to calculate and generate the answer. There are two types of informations. The first one is a static information, a word or number which don’t change everytime you reload the challange page and the second one is variable information, a word or number which changes. So we first have to open the challange page multible times and analyse the informations we need. If our script needs a static information, just copy it and save it in a variable or constant in our script, so our script don’t have to search for it, which saves us time. Getting a variable information can be difficult, because we don’t know, what we are searching for. But one thing we know…we know where it is located. The challange description is static and only the variable informations inside it are changing, so we first have to isolate the description part of the pages sourcecode, which is normally located in a div-tag without “name” or “id” parameters. Because it has no “name” or “id” we can’t directly access it with javascripts “getElementById()” or “getElementsByName()” functions, which would save us a lot time, so we have to use “getElementsByTagName()” to get every div-element and look, if it is the one, we are searching for. To do that, we have to step through every div-element, what we make with a for-loop. For that, we have to know how many div-elements we have to check, so we use the “length” function to count them. It is faster to start with the last div-element and step through them, until we reach the first one, so we do that. To check, if our current element contains only the description part, we get a string, containing the code between the open and close tag, with the “innerHTML” function and look with the “search()” function if the string start with the first words of the mission description. When we found the right element, we save our string containing the challange description and stop the for-loop with the “break” command.
This should look like the following lines, if we are searching for a challange description with starts with “Your goal is to…”:
01: var elements = document.getElementsByTagName(‘div’); //get every DIV-element 02: for (var i = elements.length - 1; i >= 0; i–) { //step through each DIV from bottom to top 03: if(elements[i].innerHTML.search(/Your goal is/) != -1 ) { //searches the elements sourcecode. If it doesn’t contain “Your goal is” it will return “-1”. So if it doesn’t return “-1” we have found it 04: var description = elements[i].innerHTML; //save our challange description 05: break; //stop the for-loop 06: } 07: }
After we found our challange description, we have to filter out our variable informations. There are 2 ways to do this. We can cut them out of the challange description with the “substr()” and “substring()” functions or we can search for it with the “match()” function. Cut them out always works and the way with the match-function only works in specific cases.
0.2.3.1) The Match-Function
Lets start with the match-function… If we want to get a specific word or number out of a string, we use the match-function, which checks all characters and words of the string and compares them with a RegExp-rule. If a part of the string fits the rules, this part will be returned by the function. Here some examples:
Our example string is: var string = “The number 1234 is even, 1235 not!”;
Example 1: var finds = string.match(//d/); finds -> “1” This will search for the 1st digit and save it in finds.
Example 2: var finds = string.match(//d+/); finds -> “1234” This will search for the 1st number and save it in finds.
Example 3: var finds = string.match(//d+/g); finds[0] -> “1234”, finds[1] -> “1235” This will search for all numbers and save them in finds.
So if we only need to find out a number or digit, the match-function is a good way to get it. Note that the numbers could be saved as strings, so use “parseInt()” to cast them to integers, before you calculate with them.
0.2.3.2) The Substr- and Substring-Functions
The other way is to cut the variable information out of the challange description. We do that with “substr()” and “substring()”. This is a very easy way, if the variable information has always the same length(like the Hash in Timed 3 which has always 32 characters), because the positions we need to cut it out never changes. Here is an example:
Example string: var string = “The hash 4d5257e5acc7fcac2f5dcd66c4e78f9a is unreversible”;
Example 1: var hash = string.substr(9,32); hash -> “4d5257e5acc7fcac2f5dcd66c4e78f9a” The substr-function starts cutting at position 9(this is the 10th character because the positions starts with 0) and cuts out the next 32 characters.
Example 2: var hash = string.substring(9,42); hash -> “4d5257e5acc7fcac2f5dcd66c4e78f9a” The substring-function starts cutting at position 9 and stops cutting at position 42.
It’s more difficult if the length of the variable information isn’t fixed. Let us start with an example. Our challange description is “What is 1 + 123? Post back the answer!”, where the number “123” is randomly generated and can be something between 1 and 999. We need both numbers to calculate the answer. The “1” is static so we know the 1st number, but to cut out the 2nd number, we need the positions where the 2nd number starts and ends or where it stats and how long it is. In this case, we know where we have to start cutting, because “What is 1 + “ at position 0 to 12 never changes, but we don’t know where to stop. But what we know is, that “? Post back the answer!” at position 12+x to 12+x+23 also never changes. So we just have to find out the position, where “? Post back the answer!” starts. To find that out we use the “search()” function again.
The code for this example is:
01: var string = “What is 1 + 123? Post back the answer!” 02: var pos = string.search(/? Post back the answer!/); 03: var 2ndnumber = string.substring(13, pos); 04: var 1stnumber = 1;
0.2.4.) Generate the answer
This is the point, which is different at all Timed Challanges. If you need help here, scroll down and look for the challenge-chapters of this article.
0.2.5.) Sending back the answer
The last thing we have to do at all challanges is to send back the answer. There are 3 ways to send it. If there is a textfield to send the answer, we automatically fill in our answer and click the sumit-button. If there is no textfield look at the challange description. If it is mentioned something like “get” or “?something=answer” we have to use the GET-method. If it’s mentioned something like “post” we have to make our own form and submit it with the POST-method.
0.2.5.1) Filling out a existing form
If there is a answer-form, we just have to insert our answer and submit it. To do that we have to find out, what are the values of the name-parameters. Just open the sourcecode of the challange page and look for the textfield and the submit button and write down their names.
Here is the code for a textfield with the name “ans” and a button with the name “submit”:
01: var ourtextfield = document.getElementsByName(“ans”)[0]; //get the first html-element with the name “ans” 02: var ourbutton = document.getElementsByName(“submit”)[0]; //get the first html-element with the name “submit” 03: ourtextfield.value = “our answer”; //change the value of the textfield into “our answer” 04: ourbutton.click(); //clicks the button to submit the form
0.2.5.2) Submitting with GET-Method
This is very easy. To submit a answer with the GET-method just open the path of the challange page and add your parameters, you want to submit, behind it. Do it this way “http://www.somedomain.com/somefile.php?param1=value1¶m2=value2¶m3=value3”.
Here is an example…
If our challenge page is “http://www.hellboundhackers.org/challenges/timed/timed5/index.php” and we want to submit the parameter “password” with the value “Our Answer” we just need this two lines:
01: var ouranswer = “Our Answer”; 02: document.location=“http://www.hellboundhackers.org/challenges/timed/timed5/index.php?password=” + ouranswer;
0.2.5.3) Submitting with POST-Method
This is probably the hardest way. If there is no form to post back our answer, we have to insert our own form into the challange page and then submit it. First we have to find a place, where we can insert our new form. I usually use the div-element which contains the challange description, because we already found it and change it, so it contains our new form.
For example we want to submit the parameter “PASSWORD” with the value “our answer” to the challange page and we saved the div-element, which contains the challange description, before in a variable called “descriptionelement”.
This should look like:
01: var ouranswer = “Our Answer”; 02: descriptionelement.innerHTML = “”;
Now there should be no challange description but our form with our answer instead. Then just submit the form with this code line:
03: ourbutton.click();
##################################################
1.) Timed Challange 1
1.1.) What we have to do
First lets see, what the description tells us to do…
“Decrypt the following random string: cnRhYH01YDFqMGF4JDEybA== and answer like this: /challenges/timed/timed1/index.php?b64=decryptedString, you have 1 second to do this in!”
OK, there is a encrypted string and we have to decrypt it. But the description doesn’t tells us (directly) which type of encryption is used. You can try some decryption algorithms but that will not give you any hints, because the decrypted string isn’t a word, it is a random full range ASCII string. Look at the “index.php?b64=decryptedString” and think of which encryption is used here. Then we use the right decryption algorithm on the string and we have our answer, which we have to submit as value of the “b64” parameter. This can be difficult, because the decrypted string can be non alphanumeric, so it’s good idea to make it URL friendly first.
1.2.) How we do it
We use a greasemonkey-script, because we only have 1 second to submit the answer. See “0.2.2.) Greasemonkey” for more informations. The first action of our script is reading the encrypted string out of the challange pages sourcecode. Read “0.2.3.) Getting the needed informations” for more help. Then we have to decrypt the string. If you are a js pro, you can code your own decryption algorithm. If you are not, just google for a working javascript ****** decryption algorithm and use it in our script. But it is not easy to find a working one, because most algorithms I have found didn’t work correctly in every case. Now we take our decrypted string and use the “escape()” function to make it URL-friendly and submit it(see “0.2.5.2) Submitting with GET-Method” for help).
##################################################
2.) Timed Challange 2
2.1) What we have to do
First lets see, what the description tells us to do…
“You will be given a randomly generated md5 hash and your task is to add up all the numbers in the md5 string. You have two seconds to answer this challenge and your string is: 8de93eaa8b2eca8725a41b1c92e488ba
The sum of all the numbers in the string is:“
This discription is very misinterpretative, so I’m telling you what we exaclty have to do…
We have to take out EVERY DECIMAL DIGIT of the random MD3-string and add these together. The sum of them is our answer, which we have to type into the textfield und submit it in less then 1 second.
An Example with the MD5-hash “8de93eaa8b2eca8725a41b1c92e488ba”:
The answer is “89” because of “8+9+3+8+2+8+7+2+5+4+1+1+9+2+4+8+8 = 89”
2.2) How we do it
And again we use a greasemonkey-script(see “0.2.2.) Greasemonkey”) to do it, because of the 1 second deadline. First our script has to extract the MD5-string out of the sourcecode of the challange page. That’s easy, because we know that MD5-hashes always consist 32 characters. Read “0.2.3.) Getting the needed informations” and “0.2.3.2) The Substr- and Substring-Functions” if you don’t know what’s to do. Next we have to extract all decimal digits out of this string and store them in an array. That isn’t difficult, just read “0.2.3.1) The Match-Function” and combine the 1st and 3rd example. Now add the digits together and submit the sum with the given form. (“0.2.5.1) Filling out a existing form”)
##################################################
3.) Timed Challange 3
3.1.) What we have to do
First lets see, what the description tells us to do…
“You will be given a randomly generated md5 hash and your task is to crack the hash using the wordlist found here and return the plaintext. You have three seconds to answer this challenge and your string is: df53ca268240ca76670c8566ee54568a If your answer is incorrect or you are out of time, you will be prohibited from retrying for five minutes.”
There is a word, we have to submit as answer, but we don’t know the word, we only have its MD5-hash. We know that a hash is the product of a one way encryption and is unreversible. So we can’t reverse it to the string it was before but how could we find out the plaintext? We have a wordlist and one of the words in it, is the one we are searching for. So we just have to code our own dictionary-md5-cracker, which steps through each word in the list, generates the MD5-hash for it and compares this hash with the hash the challange description gives us. If they are the same, we know the word we have to submit with the given form.
3.2.) How we do it
We use a greasemonkey-script, because we only have 3 second to submit the answer. See “0.2.2.) Greasemonkey” for more informations. Then we need the random MD5-hash and read it out of the challange pages sourcecode like it is written here “0.2.3.) Getting the needed informations” and there “0.2.3.2) The Substr- and Substring-Functions”. And we need the word of the wordlist. Because it’s very difficult(but possible with the XMLHttpRequest(more infos here: http://www.javascripter.net/faq/xmlhttpr.htm)) to read words out of a txt-file with javascript, we make an array which holds the words, so we can easily step through them with a for-loop.
Here is the array: var word = new Array(“12345”,“abc123”,“password”,“computer” ,“123456”,“tigger”, “1234”,“a1b2c3”,“qwerty”,“123”, “xxx”,“money”,“test”,“carmen”, “mickey”,“secret”,“summer”,“internet”, “service”,“canada”,“hello”,“ranger”,“shadow”,“baseball”, “donald”,“harley”,“hockey”,“letmein”, “maggie”,“mike”,“mustang”,“snoopy”,“buster”, “dragon”,“jordan”,“michael”,“michelle”, “mindy”,“patrick”,“123abc”,“andrew”, “bear”,“calvin”,“changeme”,“diamond”,“fuckme”, “fuckyou”,“matthew”,“miller”,“ou812”,“tiger”, “trustno1”,“12345678”,“alex”,“apple”,“avalon”, “brandy”,“chelsea”,“coffee”,“dave”,“falcon”, “freedom”,“gandalf”,“golf”,“green”,“helpme”, “linda”,“magic”,“merlin”,“molson”,“newyork”, “soccer”,“thomas”,“wizard”,“Monday”, “asdfgh”,“bandit”,“batman”,“boris”,“butthead”, “dorothy”,“eeyore”,“fishing”,“football”,“george”,“happy”,“iloveyou”, “jennifer”,“jonathan”,“love”,“marina”,“master”,“missy”, “monday”,“monkey”,“natasha”,“ncc1701”,“newpass”,“pamela”,““);
Also we need a md5-hashing-function. If we don’t want to write our own function(because this is very difficult) we google for an existing one or download a good one here: http://pajhome.org.uk/crypt/md5/
If we have all this done we can start to code our cracking function. The easiest way to do this is to make a for-loop which steps through each element of our wordsarray and then uses the md5 function to generate the hash of the word. Then use a if-condition to check if it is the right word. If we have found it, we end the for-loop with “break” and submit the word like it is written here “0.2.5.1) Filling out a existing form”.
If your code or computer are too slow you can generate a hash for every word and store them in a second array. So you only have to compare the hashes which saves a lot of time.
##################################################
4.) Timed Challange 4
4.1.) What we have to do
Here we have 2 seconds to unscrable a random word with a random length and submit it. So we have to analyse the given scramble-algorithm(which is pseudo-code and do not work exactly like the real code, but more about later), reverse it and code our own unscramble-algorithm. To understand the scramble-algorithm, look at the pseudo-code and think, how “falcon” is scrambled into “fclnoa”.
4.2.) How we do it
We use a greasemonkey-script, because we only have 2 second to submit the answer. See “0.2.2.) Greasemonkey” for more informations. Then we need the random scrambled word and read it out of the challange pages sourcecode like it is written here “0.2.3.) Getting the needed informations” and there “0.2.3.2) The Substr- and Substring-Functions”.
After that, we have to code our unscramble-algorithm.
First we need to know is the number of letters, the scrambled word( saved as SCRAMBLED) consists off and save the number as SCRAMBLEDLENGTH. Next we define a empty string-variable named UNSCRAMBLED which will hold our unscrambled word. We know, that the first letter of SCRAMBLED wasn’t changed, so it is also the first letter of UNSCRAMBLED( UNSCRABLED = UNSCRAMBLED + SCRAMBLED[0]). Also we know, that the last letter of SCRAMBLED is alwasy the 2nd letter of UNSCRAMBLED(UNSCRAMBLED = UNSCRAMBLED + SCRAMBLED[SCRAMBLEDLENGTH-1]). Now we use a for-loop, starting with the 3rd letter, and step through the letters, until we reach the previous last letter and check for every letter, if its position is odd or even. Letters at even positions don’t change, so the positions are the same in UNSCRAMBLED. Letters in SCRAMBLED at odd poitions were increased by 2, so it is the reverse in UNSCRAMBLED. Now we have unscrambled all letters exept for the last one. Here the real scramble-algorithm works differnt as the pseudo-code. If the position of the last letter of the unscrambled word was even before, the position wasn’t changed, but the 2nd letter was moved behind it, so the letter is at the previous last position in SCRAMBLED. But if the last letter of the unscrambled word was odd, it wasn’t moved foreward by 2, but by 1. So our last letter for UNSCRAMBLED isn’t the previous previous last letter of SCRAMBLED, but the previous last one.
The next step is to submit our unscrambled word. To do this, our script has to paste UNSCRAMBLED into the textfield and click the submit button. For more informations read “0.2.5.1) Filling out a existing form”.
##################################################
5.) Timed Challange 5
5.1) What we have to do
First lets see, what the description tells us to do…
A) “Your goal is to make 1386 into 1337” OK, we have to make 1386, which is a random generated number, which we call RANDOMNUMBER in this tutorial, into 1337. That should not be the problem. We only have find out what number we have to add to RANDOMNUMBER, so RANDOMNUMBER will become 1337. This number we call ADDINGNUMBER. Our formula is: 1337 - RANDOMNUMBER = ADDINGNUMBER Example: 1337 - 1386 = -49
B) “and submit how you did it: plus_num, or minus_num” What means “how you did it”? That means what number you have to add, so RANDOMNUMBER will become 1337. So you have to submit ADDINGNUMBER as answer, but instead of the sign(+ or -) in front of the number, you write “plus_” or “minus_” in front of it. If our ADDINGNUMBER would be -49, we have to submit “minus_49”.
C) “using ?password=answer, where “answer” = plus_num, or minus_num” “Using ?password=answer”…how should we submit our answer? “?password=” is the key here…if you still don’t know what’s to do here read “0.2.5.) Sending back the answer” again.
5.2) How we do it
Getting the answer for this challenge is very easy(see above). If you are smart enough, you could get it with mental arithmetic in some seconds and submit it by hand. The problem here is, that we only have 1 or 2 seconds to submit the right answer. So using a Greasemonkey-script is an good idea, because it can read the needed numbers, generate the answer and submit it in below 1 second. We just have to open the challenge and get the 30 points…there is only one problem…we have to program it first ;)
We install Firefox and Greasemonkey, if we don’t already have them. Then we open our javascript editor or notepad and start programing!
*** Note: I start the codelines with “XX: “, where “XX” is the number for the line, so dont use the linenumber information in your script! ***
5.2.1.) Writing the Greasemonkey-script-header
Ok, lets start writing our script. First we have to write our Greasemonkey header.
Look at “0.2.2.1) The Greasemonkey-Header”. This header works for our script, so use it, if you dont want to change something.
5.2.2.) Programing the Javascript
We now have to program the 2nd logical part of our Greasemonkey-script. We can use special Greasemonkey-functions, but we dont need them for our script, so we just code with normal javascript below the Greasemonkey-header. Our javascript consists of 4 locical parts, which are…
5.2.2.1.) Getting the RANDOMNUMBER
This is probably the hardest part of this challenge. We have to analyse the sourcecode of index.php and extract the RANDOMNUMBER. The problem is, that we dont know, what this number is. So we can’t directly search for it, but we know, where it is…so look into the sourcecode of index.php and find the html tag which holds the “Your goal is to make” directly at the beginning, which is direct in front of RANDOMNUMBER, which we are trying to get. If we found the right tag, which starts with “Your goal is to make”, we know the first number is RANDOMNUMBER, so we can search for it with RegExp “//d+/”. This will find the first number in a string.
Your code should do the following things: 8: get every div-tag //Because one of this tags holds the information we want to get 9: count the elements of the array which holds every div-tag //we need this information for our for-loop 10: make a for-loop, to step through every div tag //start with the last found div-tag, so we find the right one earlyer 11: get a string, which hold the htmlcode which is between the “” and “” //we have to search for a string in it next 12: make an if-condition which checks, if our string from line 11 starts with “Your goal is” //if the condition is true we know, that we have the right div-tag and RANDOMNUMBER is the first number in that string 13: if the condition is true, we read the first number out of the string, we got in line 11 //We find out what our RANDOMNUMBER is 14: we have what we want, so we break the for-loop 15: close the if-condition 16: close the for-loop
We should have our RANDOMNUMBER now and 1337 is static, so we know both numbers. Lets go and use them to calculate the ADDINGNUMBER.
5.2.2.2.) Calculating the ADDINGNUMBER
I thinks its clear, what to do here…
Your code should do the following things: 17: in line 13 we got RANDOMNUMBER as a string, so parse it from string to integer // I got some problems by calculating with integers and strings so parse it to an integer first 18: substact 1337 with RANDOMNUMBER and you will get ADDINGNUMBER //Remember what i have said in “##### What we have to do ######”
5.2.2.3.) Changing the sign to “plus_” or “minus_”
I thinks its also clear, what to do here…
Your code should do the following things: 19: make an if-condition and check if ADDINGNUMBER is higher then “0”. //because we want to find out if ADDINGNUMBER is a positive or negative number 20: if ADDINGNUMBERr is positive, we make a variable called “ANSWER” and set its value to “plus_”+ADDINGNUMBER //we now have our answer string for a positive ADDIGNNUMBER 21: make an “else” 22: we make ADDINGNUMBER positive //because if we would concat “minus_” with ADDINGNUMBER(which is for example -51), we would get “minus_-51” but we want “minus_51” 22: if ADDINGNUMBER is negative, we make a variable called “ANSWER” and set its value to “minus_”+ADDINGNUMBER //we now have our answer string for a negative ADDIGNNUMBER 23: end the if-comparison
5.2.2.4.) Submiting the answer
We don’t need to encode the answer, so just submit the answer.
Your code should do the following things: 24: open a the same page you are(index.php), but with “?password=ANSWER” behind it //because we want to submit the parameter “password” with the value ANSWER(our answer string from line 20 or 22)
Our script should be ready to use now. Save it as timed5.user.js and drag that file into your firefox and start the challenge. If your script works, you get 30 Points in under 1 second! :)
5.3.) Tips
-Code your script step by step and test it often, so you see earlier, whats wrong.
-Usefull js functions/elements for this challenge are: “document.location”, “.innerHTML”, “document.getElementsByTagName()”, “parseInt()”, “.search()”, “Math.abs()” and “.match()”
##################################################
8.) Timed Challange 8
8.1) What we have to do
First lets see, what the description tells us to do…
“You have to find out the value of ‘a’. Post back your answer, where ans = a
a+37x29=215“
OK, I think I don’t have much to expain here. We have only 1 or 2 seconds to read 3 numbers out of the sourcecode of the challange page, then do some simple arithmetics with them and POST back the number, which is our answer, as as a value for the “ans” parameter.
8.2) How we do it
We code a greasemonkey-script again(see “0.2.2.) Greasemonkey”). We reload the challange page multible times and see, that the 3 numbers and their length are changing. Read the “0.2.3.) Getting the needed informations” and you should know, how to read them with our script. Then we need to find out what “a” is, which is very easy. Only remeber that you have to multiply and devide, before you starting to add or substract something. After you have “a” you only have to POST it to the challange page. Read “0.2.5.3) Submitting with POST-Method” if you dont know how to do that.
##################################################
9.) Epilogue
This is my first article and English isn’t my native language, so please PM me, if something is wrong. If this article helped you, please vote it.
Have Fun with these challanges!
Dunuin
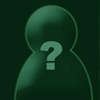
clone4 16 years ago
It's good article, but too narrow. Why you did just timed 5? Maybe introduce Greasemonkey more in depth, code snippets, explanations, essential functions, and then roughly go through all the timed challenges, and introduce what will be needed. So add more stuff and then it's gonna be superb article ;)
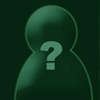
Dunuin 16 years ago
ok, I have updated my article now. It goes a little more into the deep. Perhaps I will add the other timed challenges later.
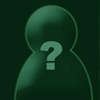
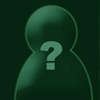
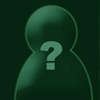
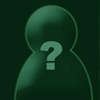
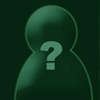
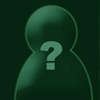
ghost 15 years ago
var b64 = atob(document.getElementsByTagName('div')[31].innerHTML.substring(47,71); document.location += 'b64=' + b64;
timed 1
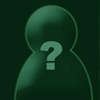
ghost 15 years ago
timed challenge 3 is a lot harder than it has to be in this article. a map of all the value is here: http://pastebin.com/f7b641735
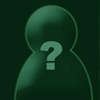
tkearn5000 15 years ago
sunglasses, your massive spoiler is missing a parenthesis, and the indices are wrong. Oh, and its a huge spoiler. Other than that, great article.
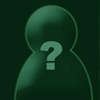
kronos666 14 years ago
i need some help please…how do i define the location of the b64 string withing the code of the page? i have the js code but the b64 string is random so i have to point the js code to read from a location.