AJAX Tutorial
AJAX Tutorial
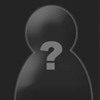
###Asynchronous Javascript and XML### A look at how AJAX works
This tutorial will show you how ajax works and how to use it from a web developers standpoint. Ever wonder how things like G-Talk work? they don’t reload the page or use Iframes and yet they always appear to have the data thats coming in instantly. it acomplishes this using ajax. Ajax is not a language of its own, so if you know javascript and HTML, your all set.
This is where it all comes from, the ability to send and recieve data without reloading the page. First, you need to create an object though,
Firefox: var xmlhttp=new XMLHttpRequest; IE: xmlhttp=new ActiveXObject(“Microsoft.XMLHTTP”);
The best way I have found to get them both in one command is:
xmlhttp=window.XMLHttpRequest?new XMLHttpRequest():new ActiveXObject(“Microsoft.XMLHTTP”);
Now you need to create a callable function as well as an onreadystatechange handler. This part is simple. Here’s an example:
function getnamedata() { xmlhttp.open(“POST”,“file.php”); xmlhttp.onreadystatechange=handler; xmlhttp.send(“name=random&id=42”); }
function handler() { if(xmlhttp.readyState==4) { alert(“Name Info: “+xmlhttp.responseText; xmlhttp.close; } }
The xmlhttp.open call opens a connection to the page, the first parameter is POST or GET depending on the method you want to use. The second parameter is the filename of the page relative to the current directory. Line 2 tells it that whenever it goes through a statechange to call a certian function, the handler. xmlhttp.send passes variables to the script that you are calling, you’ll notice that it passes them in almost exactly the same way as a url bar minus the ‘?’.
The handler function gets called every time that xmlhttp changes state. We have the if statement so that it will only execute when readyState==4 (4 is completed). The last statement of course retreives the data from the xmlhttp object. If you are getting most types of data you should use the responseText property, for actual XML data use the responseXML property. The last statement just closes the socket for later use.
Using this as a basic model you can make an entire website live if you want to, or just find something fun to do with it.
–download,
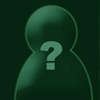
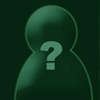
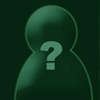
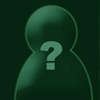
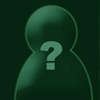
ghost 16 years ago
Very nice. Finally, something easy enough I can understand it, but still informative.