Welcome to HBH! If you have tried to register and didn't get a verification email, please using the following link to resend the verification email.
Python word unscrambler - Python Code Bank
Python word unscrambler
Unscrambles jumbled up words. Code's a bit messy. Coded by: southafrica1
#!/usr/bin/python
# Filename: unscramble.py
###########################################################################################################################
# Python version: 2.6.2
# written by: southafrica1
# email: scottkriel@yahoo.com
# Published on www.hellboundhackers.org
# Used to unscramble words which have had their letters swopped around
#
# I wrote this when I first learned python, so the code's a bit messy and there's lots of optimization that can be done
# Note: The program has a few problems when double letters appear. Modify the code and see if you can fixthat problem
# Let me know if you optimize the code. I'd like to see it cleaned up a bit
###########################################################################################################################
same = [] # setup some lists to be used later
double = []
def unscramble(string):
f = open('dictionary.txt', 'r') # This is the wordlist you want to use
running = True
while True:
line = f.readline()
if len(line) == 0: # Breaks the loop when it reaches the end of the wordlist
break
if (string[:1]) in (line): # Starts checking each character in your scrambled
aletter1 = 0 # word seperately against each word in the wordlist
else: # and gives it the value of 0 if it appears in that word
aletter1 = 1
if (string[1:2]) in (line):
aletter2 = 0
else:
aletter2 = 1
if (string[2:3]) in (line):
aletter3 = 0
else:
aletter3 = 1
if (string[3:4]) in (line):
aletter4 = 0
else:
aletter4 = 1
if (string[4:5]) in (line):
aletter5 = 0
else:
aletter5 = 1
if (string[5:6]) in (line):
aletter6 = 0
else:
aletter6 = 1
if (string[6:7]) in (line):
aletter7 = 0
else:
aletter7 = 1
if (string[7:8]) in (line):
aletter8 = 0
else:
aletter8 = 1
if (string[8:9]) in (line):
aletter9 = 0
else:
aletter9 = 1
if (string[9:10]) in (line):
aletter10 = 0
else:
aletter10 = 1
awhole = (aletter1 + aletter2 + aletter3 + aletter4 + aletter5 + aletter6 + aletter7 + aletter8 + aletter9 + aletter10) # This adds the values of all the characters together
if len(line) == len(string) + 1:
if (awhole) == 0: # If awhole = 0 then all the characters in the string appear in the word of the list
same.append(line.strip()) # Appends the word to the list we setup in the beginning
running = True # This part then checks the characters in the list of words against the string.
for i in range(0, (len(same))): # By doing this we check that all the characters in the list appear in the string.
# If we didn't do this the program would say that 'school' = 'slouch' because all the characters in 'school' appear in 'slouch',
while True: # but not all the characters in slouch appear in school.
if (same[i][:1]) in (string):
bletter1 = 0
else:
bletter1 = 1
if (same[i][1:2]) in (string):
bletter2 = 0
else:
bletter2 = 1
if (same[i][2:3]) in (string):
bletter3 = 0
else:
bletter3 = 1
if (same[i][3:4]) in (string):
bletter4 = 0
else:
bletter4 = 1
if (same[i][4:5]) in (string):
bletter5 = 0
else:
bletter5 = 1
if (same[i][5:6]) in (string):
bletter6 = 0
else:
bletter6 = 1
if (same[i][6:7]) in (string):
bletter7 = 0
else:
bletter7 = 1
if (same[i][7:8]) in (string):
bletter8 = 0
else:
bletter8 = 1
if (same[i][8:9]) in (string):
bletter9 = 0
else:
bletter9 = 1
if (same[i][9:10]) in (string):
bletter10 = 0
else:
bletter10 = 1
if (same[i][10:11]) in (string):
bletter11 = 0
else:
bletter11 = 1
if (same[i][11:12]) in (string):
bletter12 = 0
else:
bletter12 = 1
if (same[i][12:13]) in (string):
bletter13 = 0
else:
bletter13 = 1
if (same[i][13:14]) in (string):
bletter14 = 0
else:
bletter14 = 1
if (same[i][14:15]) in (string):
bletter15 = 0
else:
bletter15 = 1
bwhole = (bletter1 + bletter2 + bletter3 + bletter4 + bletter5 + bletter6 + bletter7 + bletter8 + bletter9 + bletter10 + bletter11 + bletter12 + bletter13 + bletter14 + bletter15)
if (bwhole) == 0:
double.append(same[i])
break
running = True
while True:
scramble = raw_input("\nEnter word you would like unscrambled. Type '/end/' to quit: ")
unscramble(scramble)
print(double)
del(same[:]) # Clears the lists in preperation to unscramble the next word
del(double[:])
if scramble == '/end/': # Allows user to exit loop
break
Comments
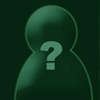
ghost 14 years ago
This code is useful for HTS Programming mission 1 isn't it???By the way it is great….Thanks for shearing :D:D:D:D:D:D:D