Brute String Generator - Python Code Bank
#!/usr/bin/env python
import sys
#copyleft by cb0 (2010) - bruteStringer.py
#first posted on HBH
########################################################################################
#Brute String Generator
#Start it with ./brutestringer.py 4 6 "abcdefghijklmnopqrstuvwxyz1234567890" ""
#will produce all strings with length 4 to 6 and chars from a to z and numbers 0 to 9
#You need to edit these settings to fit your needs.
#
#e.g. (1) When the prefix is know, use it as 4th parameter,
#You know the pass is "MyPass***" and the *** are some numbers then call it with
#./brutestringer.py 3 3 "1234567890" "MyPass"
#
#e.g. (2) ./brutestringer.py 2 3 "abc" ""
#aa
#ab
#ac
#[...]
#ccb
#ccc
########################################################################################
def rec(w, b, p):
for c in sys.argv[3]:
if (b < w - 1):
rec(w, b + 1, p + "%c" % c)
print p
for b in range(int(sys.argv[1])+1, int(sys.argv[2]) + 2):
rec(b, 0, sys.argv[4])
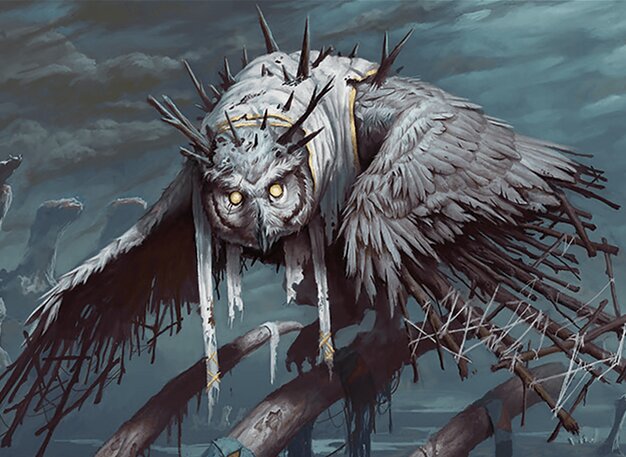
tjaart 8 months ago
Here is an updated version of the code:
#!/usr/bin/env python3 import sys import argparse
def generate_combinations(length, current_depth, prefix, charset, file_handle): “”“ Recursively generates all combinations of strings of a specified length using the provided charset and writes them to the file. “”“ for char in charset: if current_depth < length - 1: # Continue building the string generate_combinations(length, current_depth + 1, prefix + char, charset, file_handle) else: # Write the completed string to the file file_handle.write(prefix + char + ‘\n’)
def main(): # Parse command-line arguments parser = argparse.ArgumentParser(description=“Brute-force string generator.”) parser.add_argument(‘min_length’, type=int, help=“Minimum length of the generated strings.”) parser.add_argument(‘max_length’, type=int, help=“Maximum length of the generated strings.”) parser.add_argument(‘charset’, type=str, help=“Character set to use for string generation.”) parser.add_argument(‘prefix’, type=str, help=“Prefix to prepend to all generated strings.”) parser.add_argument(‘output_file’, nargs=’?’, default=‘output.txt’, help=“Output file to store generated strings. Defaults to ‘output.txt’.”)
args = parser.parse_args()
# Validate min_length and max_length
if args.min_length <= 0 or args.max_length < args.min_length:
print("Error: Ensure that min_length > 0 and max_length >= min_length.")
sys.exit(1)
# Begin the string generation process
try:
with open(args.output_file, 'w') as file_handle:
print(f"Generating strings of length {args.min_length} to {args.max_length}...")
print(f"Using charset: {args.charset}")
print(f"Prefix: '{args.prefix}'")
print(f"Output will be saved to: {args.output_file}")
print("Generation started...")
for length in range(args.min_length, args.max_length + 1):
generate_combinations(length, 0, args.prefix, args.charset, file_handle)
print("Generation completed successfully.")
except IOError as e:
print(f"Error: Unable to write to file '{args.output_file}'. {e}")
sys.exit(1)
if name == “main”: main()