Welcome to HBH! If you have tried to register and didn't get a verification email, please using the following link to resend the verification email.
Face Recognition - Python Code Bank
Face Recognition
I have facial recognition working thanks to fdlib.dll
I haven't mapped it to the mouse yet, and only supports only 1 face at the moment.
#fdtest.cpp mostly ported to python
#supports 1 face detection so far
#http://www.kyb.mpg.de/bs/people/kienzle/facedemo/facedemo.htm
#Tech B.
#3/9/2010
from ctypes import *
import Image, os
#add style and clear screen
os.system('color 02')
os.system('cls')
fd = cdll.LoadLibrary("fdlib.dll") #dll found at http://www.kyb.mpg.de/bs/people/kienzle/facedemo/facedemo.htm
im = Image.open(raw_input("Image to load: "))
#converts to grayscale if not already
if im.mode != 'L':
im = im.convert("L")
#assignments
pixX = 0
pixY = 0
pixList = []
graydata = c_ubyte * (640*480)
graydata = graydata()
w = c_int(640)
h = c_int(480)
threshold = c_int(0)
x = c_int * 256
y = c_int * 256
size = c_int * 256
x = x()
y = y()
size = size()
#obtain list of pixels
#this is an expensive algarythem, takes too long to complete...
while pixX*pixY <= (640*480):
pixList.append(im.getpixel((pixX,pixY)))
pixX += 1
if pixX == 640:
pixX = 0
pixY += 1
if pixY == 480:
break
#convert pixel list from above to ctype array
count = 0
while count < (640*480):
graydata[count] = c_ubyte(pixList[count])
count += 1
print "\nDetecting faces...\n"
fd.fdlib_detectfaces(byref(graydata), w, h, threshold)
n = fd.fdlib_getndetections()
if n == 1:
print "%d face found\n" % n
fd.fdlib_getdetection(c_int(0), x, y, size)
print "X: %d Y: %d Size: %d" % (x[0], y[0], size[0])
Comments
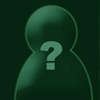
techb 14 years ago
This is the updated version. /works in real time via webcam and pygame. Can also detect multiple faces. Requires: PIL Pygame fdlib.dll VideoCapture Pyscho
#Real time face detection.
#supports 1 face detection so far
#http://www.kyb.mpg.de/bs/people/kienzle/facedemo/facedemo.htm
#Tech B.
#3/10/2010
from VideoCapture import Device
from ctypes import *
import Image, ImageDraw, os, time, pygame, sys
from pygame.locals import *
from psyco import full
full()
#add style and clear screen
os.system('color 02')
fd = cdll.LoadLibrary("fdlib.dll") #dll found at http://www.kyb.mpg.de/bs/people/kienzle/facedemo/facedemo.htm
#assignments
cam = Device()
pygame.init()
screen = pygame.display.set_mode((640,480))
pygame.display.set_caption('Facial Recognition')
pixX = 0
pixY = 0
pixList = []
w = c_int(640)
h = c_int(480)
threshold = c_int(0)
x = c_int * 256
y = c_int * 256
size = c_int * 256
x = x()
y = y()
size = size()
graydata = c_ubyte * (640*480)
graydata = graydata()
while 1:
for event in pygame.event.get():
if event.type == pygame.QUIT: sys.exit()
im = cam.getImage()
draw = ImageDraw.Draw(im)
img = im.convert("L")
imd = list(img.getdata())
cnt = 0
pixX = 0
pixY = 0
cnt = 0
for pix in imd:
graydata[cnt] = imd[cnt]
cnt+=1
fd.fdlib_detectfaces(byref(graydata), w, h, threshold)
n = fd.fdlib_getndetections()
if n == 1:
fd.fdlib_getdetection(c_int(0), x, y, size)
bBoxTres = size[0]/2
draw.rectangle([(x[0]+bBoxTres,y[0]+bBoxTres),(x[0]-bBoxTres,y[0]-bBoxTres)], outline=255)
imn = pygame.image.frombuffer(im.tostring(), (640,480), "RGB")
screen.blit(imn, (0,0))
pygame.display.flip()
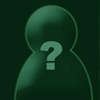
techb 14 years ago
This is the updated version. /works in real time via webcam and pygame. Can also detect multiple faces. Requires: PIL Pygame fdlib.dll VideoCapture Pyscho
#Real time face detection.
#supports 1 face detection so far
#http://www.kyb.mpg.de/bs/people/kienzle/facedemo/facedemo.htm
#Tech B.
#3/10/2010
from VideoCapture import Device
from ctypes import *
import Image, ImageDraw, os, time, pygame, sys
from pygame.locals import *
from psyco import full
full()
#add style and clear screen
os.system('color 02')
fd = cdll.LoadLibrary("fdlib.dll") #dll found at http://www.kyb.mpg.de/bs/people/kienzle/facedemo/facedemo.htm
#assignments
cam = Device()
pygame.init()
screen = pygame.display.set_mode((640,480))
pygame.display.set_caption('Facial Recognition')
pixX = 0
pixY = 0
pixList = []
w = c_int(640)
h = c_int(480)
threshold = c_int(0)
x = c_int * 256
y = c_int * 256
size = c_int * 256
x = x()
y = y()
size = size()
graydata = c_ubyte * (640*480)
graydata = graydata()
while 1:
for event in pygame.event.get():
if event.type == pygame.QUIT: sys.exit()
im = cam.getImage()
draw = ImageDraw.Draw(im)
img = im.convert("L")
imd = list(img.getdata())
cnt = 0
pixX = 0
pixY = 0
cnt = 0
for pix in imd:
graydata[cnt] = imd[cnt]
cnt+=1
fd.fdlib_detectfaces(byref(graydata), w, h, threshold)
n = fd.fdlib_getndetections()
if n == 1:
fd.fdlib_getdetection(c_int(0), x, y, size)
bBoxTres = size[0]/2
draw.rectangle([(x[0]+bBoxTres,y[0]+bBoxTres),(x[0]-bBoxTres,y[0]-bBoxTres)], outline=255)
imn = pygame.image.frombuffer(im.tostring(), (640,480), "RGB")
screen.blit(imn, (0,0))
pygame.display.flip()