Welcome to HBH! If you have tried to register and didn't get a verification email, please using the following link to resend the verification email.
Mouse mover - Java Code Bank
Mouse mover
Moves the mouse back and fourth to prevent screen sleeping.
see: http://wiki.tak11.info/index.php?title=Mouse_Mover
package info.tak11.mousemover;
import java.awt.AWTException;
import java.awt.MouseInfo;
import java.awt.Robot;
public class MouseMover {
private static Robot mouse;
private static int[][] locations;
public static void main(String[] args) throws AWTException {
mouse = new Robot(); //create Robot object
locations = new int[2][2];
locations[0][0] = MouseInfo.getPointerInfo().getLocation().x;
locations[0][1] = MouseInfo.getPointerInfo().getLocation().y;
locations[1][0] = MouseInfo.getPointerInfo().getLocation().x + 100;
locations[1][1] = MouseInfo.getPointerInfo().getLocation().y;
while(true) {
if( !(MouseInfo.getPointerInfo().getLocation().x == locations[0][0]
&& MouseInfo.getPointerInfo().getLocation().y == locations[0][1]
|| MouseInfo.getPointerInfo().getLocation().x == locations[1][0]
&& MouseInfo.getPointerInfo().getLocation().y == locations[1][1]) ) {
System.exit(1); //they moved the mouse, were quiting
}
try {
Thread.sleep(3 * 1000);
} catch (InterruptedException e) {
e.printStackTrace();
} //sleep 3 seconds
mouse.mouseMove(MouseInfo.getPointerInfo().getLocation().x + 100, MouseInfo.getPointerInfo().getLocation().y);
try {
Thread.sleep(3 * 1000);
} catch (InterruptedException e) {
e.printStackTrace();
} //sleep 3 seconds
mouse.mouseMove(MouseInfo.getPointerInfo().getLocation().x - 100, MouseInfo.getPointerInfo().getLocation().y);
}
}
}
Comments
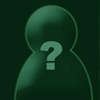
fuser 14 years ago
well, it's nice to see that it works. I think you can make an exe and get it to run every 5 mins, since in most cases people are gonna be away for more than 5 mins.
still, good job.