Welcome to HBH! If you have tried to register and didn't get a verification email, please using the following link to resend the verification email.
Webcam face detection and tracking - Java Code Bank
Webcam face detection and tracking
Tracks faces via webcam feed
/*
Requires the processing and OpenCV libraries to be installed.
Processing - http://processing.org/download/
OpenCV - http://ubaa.net/shared/processing/opencv/
*/
import processing.core.*;
import processing.xml.*;
import hypermedia.video.*;
import java.applet.*;
import java.awt.*;
import java.awt.image.*;
import java.awt.event.*;
import java.io.*;
import java.net.*;
import java.text.*;
import java.util.*;
import java.util.zip.*;
import java.util.regex.*;
public class facetrack extends PApplet {
OpenCV opencv;
int contrast_value = 0;
int brightness_value = 0;
public void setup() {
size( 320, 240 );
opencv = new OpenCV( this );
opencv.capture( width, height );
opencv.cascade( OpenCV.CASCADE_FRONTALFACE_ALT );
// print usage
println( "Drag mouse on X-axis inside this sketch window to change contrast" );
println( "Drag mouse on Y-axis inside this sketch window to change brightness" );
}
public void stop() {
opencv.stop();
super.stop();
}
public void draw() {
opencv.read();
opencv.convert( GRAY );
opencv.contrast( contrast_value );
opencv.brightness( brightness_value );
Rectangle[] faces = opencv.detect( 1.2f, 2, OpenCV.HAAR_DO_CANNY_PRUNING, 40, 40 );
image( opencv.image(), 0, 0 ); noFill(); stroke(255,0,0);
for( int i=0; i<faces.length; i++ ) {
int[] centerPoint = {faces[i].x + (faces[i].width/2), faces[i].y + ((faces[i].height/2))};
ellipse(centerPoint[0], centerPoint[1], 15, 15);
}
}
public void mouseDragged() {
contrast_value = (int) map( mouseX, 0, width, -128, 128 );
brightness_value = (int) map( mouseY, 0, width, -128, 128 );
}
static public void main(String args[]) {
PApplet.main(new String[] { "--bgcolor=#FFFFFF", "facetrack" });
}
}
Comments
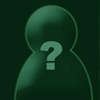