ICMP package
ICMP package
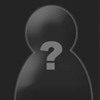
Hello, I just try here to give you an exemple on how you can make a ICMP package. Let's go !
-1- You need a structure for your package as : struct packeticmp { struct iphdr ip; /* for the ip header / struct icmphdr icmp; / for the icmp header / char buffer[100]; / If you want to send data with your icmp package */ };
-2- You need a checksum calculator : unsigned short in_cksum(u_short *addr, int len) { register int nleft = len; register u_short *w = addr; register int sum = 0; u_short answer = 0; while (nleft > 1) { sum += *w++; nleft -= 2; } if (nleft == 1) { *(u_char *)(&answer) = *(u_char *)w ; sum += answer; } sum = (sum >> 16) + (sum & 0xffff); sum += (sum >> 16); answer = ~sum; return(answer); }
-3- Now you can start he code : /* the include / #include <stdio.h> #include <netinet/ip.h> #include <netinet/ip_icmp.h> #include <netinet/in.h> / Pour IPPROTO_RAW */ #include <sys/types.h> #include <netdb.h> #include <string.h>
/* start programme / int main() { / variable declaration */ char ipsrc[16],ipdst[16]; int type=0,code=0,num=0,idp=0,fragof=0,ttlp=0,sizepacket,sizebuffer; int i,sockets; struct packeticmp icmpp; struct sockaddr_in socketss;
/* zero setting of the package */ memset(&icmpp, 0, sizeof(struct packeticmp));
/* choose your info */ printf("IP Source =>"); bzero(ipsrc,sizeof(ipsrc)); scanf("%s",ipsrc);
printf("IP Destination =>"); bzero(ipdst,sizeof(ipdst)); scanf("%s",ipdst);
printf("IP id (number of the package) =>"); scanf("%d",&idp);
printf("IP FragOff (split up ?) =>"); scanf("%d",&fragof);
printf("IP ttl =>"); scanf("%d",&ttlp);
printf("TYPE icmp (0,3,4,5,8,11 to 18) =>"); scanf("%d",&type);
printf("CODE icmp =>"); scanf("%d",&code);
printf("Number of package =>"); scanf("%d",&num);
printf("Data:"); bzero((icmpp.buffer),sizeof(icmpp.buffer)); scanf("%s",icmpp.buffer);
/* open the socket */ if(!(sockets=socket(AF_INET, SOCK_RAW, IPPROTO_RAW)))return(-1);
/* Calculate size of the package */ sizebuffer=strlen(icmpp.buffer); sizepacket=sizeof(struct iphdr) + sizeof(struct icmphdr) + sizebuffer;
/* construction of the IP header */ icmpp.ip.version = 4; icmpp.ip.ihl = 5; icmpp.ip.tot_len = htons(sizepacket); icmpp.ip.id = htons(idp); icmpp.ip.frag_off = htons(fragof); icmpp.ip.ttl = ttlp; icmpp.ip.protocol = 1; // ICMP icmpp.ip.saddr = inet_addr(ipsrc); icmpp.ip.daddr = inet_addr(ipdst); icmpp.ip.check = in_cksum((u_short *)&icmpp,sizeof(struct iphdr));
/* construction of the ICMP header */ icmpp.icmp.type = type; icmpp.icmp.code = code; icmpp.icmp.checksum = in_cksum((u_short *)&icmpp,sizeof(struct iphdr) + sizeof(struct icmphdr)+ sizebuffer);
/* info for the sendto */ socketss.sin_family = AF_INET; socketss.sin_addr.s_addr = icmpp.ip.daddr;
/* send package */ for(i=1;i<=num;i++) { if(sendto(sockets,&icmpp, sizepacket, 0, (struct sockaddr *)&socketss,sizeof(struct sockaddr)) != -1) { printf("Package ICMP n°%d go!!",i); } else { printf("Package ICMP n°%d Problem",i); perror("Erreur"); close(sockets); exit(1); } }
/* close the socket */ close(sockets); return 0; }
I hope this article can help someone. Please rate and comment thx.
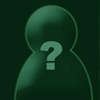
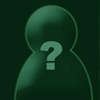
ghost 18 years ago
I don't need to look on google for know what is a icmp package lol. I'm make this (two years ago) with the RFC and all the .h in linux lol
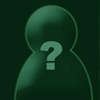
SySTeM 18 years ago
Nice article!
[url][mail][img][b]:);):|:(:o:pB):D:@:angry::happy::ninja::radio::whoa::wow::vamp::right::love::matey::evil:[/img][/mail][/b][/url]